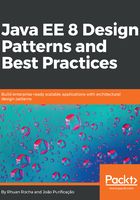
Implementing the PersistenceManager class
We need to manage all processes of persistence and query data. For this, we need to create a class.
The PersistenceManager class is responsible for managing all persistence processes and query processes:
import javax.annotation.PostConstruct;
import javax.inject.Inject;
import java.util.LinkedHashSet;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
public class PersistenceManager {
private Set<StageManager> stateManagers;
@Inject @Transactional Transaction transaction;
@PostConstruct
public void init(){
stateManagers = new LinkedHashSet<StageManager>();
}
public Optional<Entity> persist ( Entity entity ) {
if ( entity instanceof Employee ){
stateManagers.add( new EmployeeStageManager( (Employee)
entity ) );
}
return Optional.ofNullable(entity);
}
public void begin() throws Exception {
if( !transaction.isOpened() ){
transaction.begin();
}
else{
throw new Exception( "Transaction already is opened" );
}
}
public Optional<Entity> load(Object id){
Entity entity = stateManagers.stream()
.filter( e-> e.getEntity().getId().equals( id ) )
.map( s->s.getEntity() )
.findFirst()
.orElseGet( ()-> new EmployeeStoreManager().load( id ) );
if( Optional.ofNullable(entity).isPresent()
&& stateManagers.stream().map( s->s.getEntity()
).collect( Collectors.toList() ).contains( entity ) )
stateManagers.add( new EmployeeStageManager( (Employee)
entity) );
return Optional.ofNullable(entity);
}
public void commit() throws Exception {
if( transaction.isOpened() ){
stateManagers.stream().forEach( s-> s.flush() );
transaction.commit();
}
else{
throw new Exception( "Transaction is not opened" );
}
}
public void rollback() throws Exception {
if( transaction.isOpened() ) {
stateManagers = new LinkedHashSet<StageManager>();
transaction.rollback();
}
else {
throw new Exception( "Transaction is not opened" );
}
}
}
In the preceding code, we have the PersistenceManager class. This class has the stateManagers attribute, which is a set of StateManager used to control the read and write of each object model. It also has the Transaction attribute, which is used to control the transaction lifecycle. This class also has the persist method, which is used to write the data represented by the object model; the begin method, used to begin the transaction; the load method, used to read an object model's data; the commit method, used to commit the transaction; and finally, the rollback method, which is used to roll back the transaction.