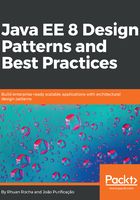
Implementing LogBrowserFilter
The implementation of LogBrowserFilter is as follows:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import javax.servlet.*;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletRequest;
import java.io.IOException;
@WebFilter(filterName = "LogBrowser",urlPatterns = "/*")
public class LogBrowserFilter implements Filter {
private static Logger logger = LogManager.getLogger(LogBrowser.class);
public void destroy() {
}
public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain) throws ServletException, IOException {
//Get userAgent that contain browse informations.
String userAgent = ((HttpServletRequest)req).getHeader("User-Agent");
//Get IP of Client that sent a resquest.
String ip = ((HttpServletRequest)req).getRemoteAddr();
//Logging the informations of IP and Browser.
logger.info("IP: "+ip +" Browser info: "+userAgent);
//Following to the next filter. If none next filter exist, follow to main logic.
chain.doFilter(req, resp);
}
public void init(FilterConfig config) throws ServletException {
}
}
In the preceding filter, we get the client IP and browser information and log them. The LogBrowserFilter operation is similar to that of LogAccessFilter.
To define the order of filter execution, we need to configure the web.xml and add the filter mapping information. Here, we can see web.xml with its configuration:
<web-app version="3.1"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd">
<filter>
<filter-name>LogBrowser</filter-name>
<filter-class>com.rhuan.filter.LogBrowserFilter</filter-class>
</filter>
<filter>
<filter-name>LogAccess</filter-name>
<filter-class>com.rhuan.filter.LogAccessFilter</filter-class>
</filter>
</web-app>
The configurations defined in web.xml override the annotation configurations. Thus, if we put the urlPattern configuration on web.xml, then the configuration considered is web.xml's configuration. We don't put the filter mapping information on web.xml because this is already on the annotation configuration in the code. The web.xml configuration defines the order—LogBrowserFilter will be called first, followed by LogAccessFilter, and then the main logic (servlet).