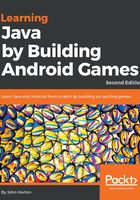
Handling different screen resolutions part 1: Initialising the variables
Add these next four (six including comments) lines of code. Study them carefully and then we can talk about them.
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Get the current device's screen resolution Display display = getWindowManager().getDefaultDisplay(); Point size = new Point(); display.getSize(size); // Initialize our size based variables // based on the screen resolution numberHorizontalPixels = size.x; numberVerticalPixels = size.y; blockSize = numberHorizontalPixels / gridWidth; gridHeight = numberVerticalPixels / blockSize; Log.d("Debugging", "In onCreate"); newGame(); draw(); }
Let's look at the first line of code because it gives us a glimpse into the later chapters of the book.
numberHorizontalPixels = size.x;
What is happening in the highlighted portion of the previous line of code is that we are accessing the x
variable contained inside the size
object using the dot operator .
.
Remember that the width of the screen in pixels has previously been assigned to x
. Therefore, the other part of the previous line numberHorizontalPixels =
initializes numberHorizontalPixels
with whatever value is in x
.
The next line of code does the same thing with numberVerticalPixels
and size.y
. Here it is again for convenience.
numberVerticalPixels = size.y;
We now have the screen resolution neatly stored in the appropriate variables ready for use throughout our game.
The final two lines does some simple math to initialize blockSize
and gridHeight
. The blockSize
variable is assigned the value of numberHorizontalPixels
divided by gridWidth
.
blockSize = numberHorizontalPixels / gridWidth;
Remember that gridWidth
was previously initialized with the value 40
. So, assuming the screen once it is made full screen and landscape (as ours has) are 1776 x 1080 pixels (as the Google Pixel emulator is) then gridWidth
will be as follows.
1776 / 40 = 44.4
Note
Actually, the Google Pixel has a horizontal resolution of 1920 but the Back, Home, and Running Apps controls take up some of the screen space.
You have probably noticed that the result contains a fraction and an int
holds whole numbers. We will discuss this further soon.
Note that it doesn't matter how high or low the resolution of the screen is blockSize
will be about right compared to the number of horizontal grid positions and when they are drawn they will fairly neatly take up the entire width of the screen.
The final line in the previous code uses blockSize
to match up how many blocks can be fitted into the height.
gridHeight = numberVerticalPixels / blockSize;
Using the resolution of the Google Pixel emulator as an example reveals the first imperfection of our code. Look at the math the previous line performs. Note that we had a similar imperfection when calculating gridWidth
.
1080 / 44 = 24.54545…
We have a floating-point (fraction) answer. First, if you remember back to our discussion of types, the .54545.. is lost leaving 24. If you look at the grid we will eventually draw you will notice the last row is a slightly different size.

This could be more or less pronounced depending upon the resolution of your chosen device/emulator.
Tip
A common mistake in understanding the float
to int
conversion is to think like we might have been taught at high school and to round the answer up or down. When a decimal fraction is placed in an int
the fractional part of the value is lost not rounded. So, it is always the lower whole number. As an extreme example, 1.999999 would become 1, not 2. When you need more accuracy or high-school rounding is required then you can do things slightly differently. We will be doing more advanced and accurate math than this as we progress through the book.
Another important point to consider for the future although it is not worth getting hung-up on now is that different devices have different ratios of width to height. It is perfectly possible, even likely that some devices will end up with a different number of grid positions than others. In the context of this project it is irrelevant but as our game projects improve throughout the book we will address these issues. In the final project, we will solve them all with a virtual camera
As the code is becoming more expansive it is more likely you will get some errors. Before we add the final code for this chapter let's discuss the most likely errors and warnings, so you know how to solve them when they arise.