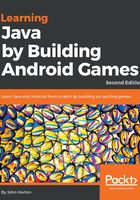
Coding the onTouchEvent method
Delete the call to takeShot
in onTouchEvent
because it was just temporary code and it needs an upgrade to handle passing some values into the method. We will upgrade the takeShot
signature to be compatible with this next code soon.
Code your onTouchEvent
method to look exactly like this next code then we will discuss it.
/* This part of the code will handle detecting that the player has tapped the screen */ @Override public boolean onTouchEvent(MotionEvent motionEvent) { Log.d("Debugging", "In onTouchEvent"); // Has the player removed their finger from the screen? if((motionEvent.getAction() & MotionEvent.ACTION_MASK) == MotionEvent.ACTION_UP) { // Process the player's shot by passing the // coordinates of the player's finger to takeShot takeShot(motionEvent.getX(), motionEvent.getY()); } return true; }
Let's look closely at the line of code that determines whether the player removed their finger from the screen.
It starts with an if(…
and at the end of the if
condition we have the body with some more code that we will look at next. It is the condition which is relevant now. Here it is unpacked from the rest of the surrounding code.
motionEvent.getAction() & MotionEvent.ACTION_MASK) == MotionEvent.ACTION_UP
First it calls motionEvent.getAction
. Remember that this is equivalent to using the returned value in the rest of the condition. The returned value is then bitwise AND with motionEvent.ACTION_MASK
. Finally, it is compared using ==
to motionEvent.ACTIONUP
.
The condition is true if the event that was triggered was equal to ACTION_UP
. This means the player has removed their finger from the screen.
Tip
You could summarize and explain the entire discussion on the MotionEvent
object and that slightly awkward line of code by saying it is all equivalent to this made-up code.
if(player removed finger);
We can now look at the code that executes when the if
statement is true. This code is much simpler to understand. Take a closer look.
// Process the player's shot by passing the // coordinates of the player's finger to takeShot takeShot(motionEvent.getX(), motionEvent.getY());
The single line of code simply calls the takeShot
method using motionEvent.getX
and motionEvent.getY
as arguments. If you remember the section Making sense of screen touches, then you know that both are float
values.
Currently, the takeShot
method takes no parameters. In the next section, we will change the takeShot
signature to accommodate these two float
values and we will also add all the code needed to process the player taking a shot.
We are so close to the finish.