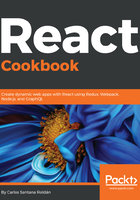
上QQ阅读APP看书,第一时间看更新
C3.js chart – implementing componentDidUpdate
C3.js is a third-party library that makes it easy to generate D3-based charts by wrapping the code required to construct the entire chart. That means you don't need to write any D3 code anymore:
- componentDidUpdate: This React method is normally used to manage third-party UI elements and interact with the native UI. When we use a third-party library such as C3.js, we need to update the UI library with the new data. Install C3.js with npm:
npm install c3
- After we install C3.js, we need to add the C3 CSS file to our index.html. For now, we can use the CDN they provide:
<!-- Add this on the <head> tag -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.4.10/c3.min.css" rel="stylesheet" />
File: public/index.html
- Now we can create our Chart component:
import React, { Component } from 'react';
import c3 from 'c3';
import './Chart.css';
class Chart extends Component {
componentDidMount() {
// When the component mounts the first time we update
// the chart.
this.updateChart();
}
componentDidUpdate() {
// When we receive a new prop then we update the chart again.
this.updateChart();
}
updateChart() {
c3.generate({
bindto: '#chart',
data: {
columns: this.props.columns,
type: this.props.chartType
}
});
}
render() {
return <div id="chart" />;
}
}
export default Chart;
File: src/components/Chart/Chart.js
- As you can see, we are executing the updateChart method on componentDidUpdate, which is executed every time the user receives a new prop from App.js. Let's add some logic that we need in our App.js file:
import React, { Component } from 'react';
import Chart from './Chart/Chart';
import Header from '../shared/components/layout/Header';
import Content from '../shared/components/layout/Content';
import Footer from '../shared/components/layout/Footer';
import './App.css';
class App extends Component {
constructor(props) {
super(props);
this.state = {
chartType: 'line'
};
this.columns = [
['BTC', 3000, 6000, 10000, 15000, 13000, 11000],
['ETH', 2000, 3000, 5000, 4000, 3000, 940],
['XRP', 100, 200, 300, 500, 400, 300],
];
}
setBarChart = () => {
this.setState({
chartType: 'bar'
});
}
setLineChart = () => {
this.setState({
chartType: 'line'
});
}
render() {
return (
<div className="App">
<Header title="Charts" />
<Content>
<Chart
columns={this.columns}
chartType={this.state.chartType}
/>
<p>
Chart Type
<button onClick={this.setBarChart}>Bar</button>
<button onClick={this.setLineChart}>Line</button>
</p>
</Content>
<Footer />
</div>
);
}
}
export default App;
File: src/components/App.js
- Now let's add some basic styles to our Chart component:
p {
text-align: center;
}
button {
background: #159fff;
border: none;
color: white;
margin-left: 1em;
padding: 0.5em 2em;
text-transform: uppercase;
&:hover {
background: darken(#159fff, 5%);
}
}
#chart {
background: #fff;
width: 90%;
margin: 1em auto;
}
File: src/components/Chart.css
- In this case, we are creating some charts to display information about the most important cryptocurrencies today (BTC - Bitcoin, ETH - Ethereum and XRP - Ripple). This is how it should look:

This image gives you an idea of how the line charts look like
- We have two buttons to switch between chart types (bar or line). If we click on BAR, we should see this chart:

This image gives you an idea of how the bar charts look like.
- If you remove the componentDidUpdate method from the Chart component, then when you press the buttons the chart is not going to update. This is because every time we need to refresh the data, we need to call the c3.generate method, and in this case, React's componentDidUpdate method is very useful.