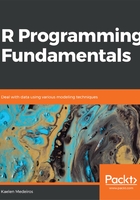
Vectors
A vector is an object that holds a collection of various data elements in R, though they are limited because everything inside of a vector must all belong to the same variable type. You can create a vector using the method c(), for example:
vector_example <- c(1, 2, 3, 4)
In the above snippet, the method c() creates a vector named vector_example. It will have a length of four and be of class numeric. You use c() to create any type of vector by inputting a comma-separated list of the items you'd like inside the vector. If you input different classes of objects inside the vector (such as numeric and character strings), it will default to one of them.
In the following code, we can see an example where the class of the vector is a character because of the B in position 2:
vector_example_2 <- c(1, "B", 3)
class(vector_example_2)
Output: The preceding code provides the following output:

To access a certain item in the vector, you can use indexing. R is a 1-indexed language, meaning all counting starts at 1 (versus other languages, such as Python, which are 0-indexed—that is, in Python, the first element of the array is said to be at the 0th position).
The first item in a vector can be accessed using vector[1], and so on. If the index doesn't exist in the vector, R will simply output an NA, which is R's default way of indicating a missing value. We'll cover missing values in R at length in Chapter 3, Data Management.
Let us now use c() to create a vector, examine its class and type, and access different elements of the vector using vector indexing. Follow the steps given below:
- Create the vectors twenty and alphabet using the following code:
twenty <- c(1:20)
alphabet <- c(letters)
- Check the class and type of twenty and alphabet using class() and typeof(), respectively, as follows:
class(twenty)
typeof(twenty)
class(alphabet)
typeof(alphabet)
- Find the numbers at the following positions in twenty using vector indexing:
twenty[5]
twenty[17]
twenty[25]
- Find the letters at the following positions in the alphabet using vector indexing:
alphabet[6]
alphabet[23]
alphabet[33]
Output: The code we write will be as follows:
twenty <- c(1:20)
alphabet <- c(letters)
class(twenty)
typeof(twenty)
class(alphabet)
typeof(alphabet)
twenty[5]
twenty[17]
twenty[25]
alphabet[6]
alphabet[23]
alphabet[33]
The output we get after executing it is as follows:
