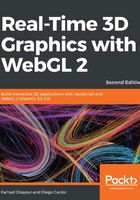
上QQ阅读APP看书,第一时间看更新
Time for Action: Querying the State of Buffers
Follow the given steps:
- Open the ch02_05_state-machine.html file in your browser. You should see the following:
- Open ch02_05_state-machine.html in your editor and scroll down to the initBuffers method:
function initBuffers() {
const vertices = [
1.5, 0, 0,
-1.5, 1, 0,
-1.5, 0.809017, 0.587785,
-1.5, 0.309017, 0.951057,
-1.5, -0.309017, 0.951057,
-1.5, -0.809017, 0.587785,
-1.5, -1, 0,
-1.5, -0.809017, -0.587785,
-1.5, -0.309017, -0.951057,
-1.5, 0.309017, -0.951057,
-1.5, 0.809017, -0.587785
];
indices = [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 5,
0, 5, 6,
0, 6, 7,
0, 7, 8,
0, 8, 9,
0, 9, 10,
0, 10, 1
];
// Create VAO
coneVAO = gl.createVertexArray();
// Bind VAO
gl.bindVertexArray(coneVAO);
const coneVertexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, coneVertexBuffer);
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices),
gl.STATIC_DRAW);
// Configure instructions
gl.vertexAttribPointer(program.aVertexPosition, 3, gl.FLOAT,
false, 0, 0);
gl.enableVertexAttribArray(program.aVertexPosition);
coneIndexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, coneIndexBuffer);
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(indices),
gl.STATIC_DRAW);
// Set the global variables based on the parameter type
if (coneVertexBuffer ===
gl.getParameter(gl.ARRAY_BUFFER_BINDING)) {
vboName = 'coneVertexBuffer';
}
if (coneIndexBuffer ===
gl.getParameter(gl.ELEMENT_ARRAY_BUFFER_BINDING)) {
iboName = 'coneIndexBuffer';
}
vboSize = gl.getBufferParameter(gl.ARRAY_BUFFER, gl.BUFFER_SIZE);
vboUsage = gl.getBufferParameter(gl.ARRAY_BUFFER,
gl.BUFFER_USAGE);
iboSize = gl.getBufferParameter(gl.ELEMENT_ARRAY_BUFFER,
gl.BUFFER_SIZE);
iboUsage = gl.getBufferParameter(gl.ELEMENT_ARRAY_BUFFER,
gl.BUFFER_USAGE);
try {
isVerticesVbo = gl.isBuffer(vertices);
}
catch (e) {
isVerticesVbo = false;
}
isConeVertexBufferVbo = gl.isBuffer(coneVertexBuffer);
// Clean
gl.bindVertexArray(null);
gl.bindBuffer(gl.ARRAY_BUFFER, null);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, null);
}
- Pay attention to how we use the methods discussed in this section to retrieve and display information about the current state of the buffers.
- The information queried by the initBuffers function is shown in the settings section of the web page when we use updateInfo.
- In the settings section of the web page, you will see the following result:
- Copy the following line, gl.bindBuffer(gl.ARRAY_BUFFER, null);, and paste it right before the following line inside of the initBuffers function: coneIndexBuffer = gl.createBuffer();.
- What happens when you launch the page in your browser again?
- Why do you think this behavior occurs?
What just happened?
You have learned that the currently-bound buffer is a state variable in WebGL. The buffer is bound until you unbind it by calling bindBuffer again with the corresponding type (ARRAY_BUFFER or ELEMENT_ARRAY_BUFFER) as the first parameter and with null as the second argument (that is, no buffer to bind). You have also learned that you can only query the state of the currently-bound buffer. Therefore, if you want to query a different buffer, you need to bind it first.