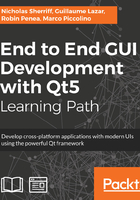
Unit testing
The essence of unit testing is to break an application down into its smallest functional blocks (units) and then test each unit with real-world scenarios within the scope of the initiative. For example, take a simple method that takes two signed integers and adds them together:
int add(intx, int y);
Some example scenarios can be as listed:
- Adding two positive numbers
- Adding two negative numbers
- Adding two zeroes
- Adding one positive and one negative number
- Adding zero and a positive number
- Adding zero and a negative number
We can write a test for each of these scenarios and then every time our code base changes (any code, not just our add() method), these tests can be executed to ensure that the code still behaves as expected. It is a really valuable tool to give you confidence that any code changes you make aren’t having a detrimental effect on the existing functionality.
Historically, these tests would have been performed manually, but tooling exists that can enable us to write code to test code automatically, which sounds like a bit of a paradox, but it really works. Qt provides a tailored framework for unit testing Qt-based applications, called Qt Test, and that is what we will use.
Test-driven development (TDD) takes unit testing to the next level and actually changes the way you write code in the first place. In essence, you write a test first. The test will initially fail (indeed, probably it won’t even build) because you have no implementation. You then write the bare minimum of code it takes to make the test pass and then move on to writing the next test. You iteratively build out your implementation in this way until you have delivered the block of functionality required. Finally, you refactor the code to the required standard, using the completed unit tests to validate that the refactored code still behaves as expected. This is sometimes referred to as Red-Green-Refactor.
This isn’t a book about unit testing, and it is certainly not about TDD, so we will be very loose with our approach, but it is a key part of modern application development, and it is important to know how it fits into your Qt projects.
We’ve demonstrated the mechanism for passing a simple piece of data (the welcome message) from our business logic project to our UI, so as always, starting as simply as possible, our first goal for this chapter is to write a rudimentary unit test for that behavior. Once done, we’ll move on to test the data classes we implemented in the previous chapter.