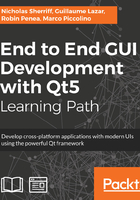
qmake
Our project (.pro) files are parsed by a utility called qmake, which in turn generates Makefiles that drive the building of the application. We define the type of project output we want, what source files are included as well as the dependencies and much more. Much of this is achieved by simply setting variables as we will do in our project file now.
Add the following to scratchpad.pro:
TEMPLATE = app QT += qml quick CONFIG += c++14 SOURCES += main.cpp RESOURCES += qml.qrc
Let’s run through each of these lines in turn:
TEMPLATE = app
TEMPLATE tells qmake what type of project this is. In our case, it’s an executable application that is represented by app. Other values we are interested in are lib for building library binaries and subdirs for multi project solutions. Note that we set a variable with the = operator:
QT += qml quick
Qt is a modular framework that allows you to pull in only the parts you need. The QT flag specifies the Qt modules we want to use. The core and gui modules are included by default. Note that we append additional values to a variable that expects a list with +=:
CONFIG += c++14
CONFIG allows you to add project configuration and compiler options. In this case, we are specifying that we want to make use of C++14 features. Note that these language feature flags will have no effect if the compiler you are using does not support them:
SOURCES += main.cpp
SOURCES is a list of all the *.cpp source files we want to include in the project. Here, we add our empty main.cpp file, where we will implement our main() function. We don’t have any yet, but when we do, our header files will be specified with a HEADERS variable:
RESOURCES += qml.qrc
RESOURCES is a list of all the resource collection files (*.qrc) included in the project. Resource collection files are used for managing application resources such as images and fonts, but most crucially for us, our QML files.
With the project file updated, save the changes.
Whenever you save a change to your *.pro files, qmake will parse the file. If all is well, you will get a small green bar at the bottom-right of Qt Creator. A red bar indicates some kind of issue, usually a syntax error. Any output from the process will be written out to the General Messages window to help you diagnose and fix the problem. White space is ignored, so don’t worry about matching up the blank lines exactly.
You will see that our other files have now magically appeared in the Projects pane:

Double-click on main.cpp to edit it, and we’ll write our first bit of code:
#include <QGuiApplication> #include <QQmlApplicationEngine>
int main(int argc, char *argv[]) { QGuiApplication app(argc, argv); QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
return app.exec(); }
All we are doing here is instantiating a Qt GUI application object and asking it to load our main.qml file. It’s very short and simple because the Qt framework does all the complex low-level work for us. We don’t have to worry about platform detection or managing window handles or OpenGL.
Possibly one of the most useful things to learn is that placing the cursor in one of the Qt objects and pressing F1 will open the help for that type. The same is true for methods and properties on Qt objects. Poke around in the help files to see what QGuiApplication and QQmlApplicationEngine are all about.
To edit the next file in our project—qml.qrc—you need to right-click and select the editor you want to open it with. The default is Resource Editor:

I am personally not a fan of this editor. I don’t feel it makes editing any easier than just writing plain text and isn’t particularly intuitive. Close this and instead choose Open with > Plain Text Editor.
Add the following content:
<RCC> <qresource prefix="/"> <file>main.qml</file> </qresource> </RCC>
Back in main.cpp, we asked Qt to load the qrc:/main.qml file. This essentially breaks down as “look for the file in a qrc file with a prefix of / and a name of main.qml”. Now here in our qrc file, we have created a qresource element with a prefix property of /. Inside this element, we have a collection of resources (albeit only one of them) that has the name main.qml. Think of qrc files as a portable filesystem. Note that the resource files are located relative to the .qrc file that references them. In this case, our main.qml file is in the same folder as our qml.qrc file. If it was in a subfolder called views, for example, then the line in qml.qrc would read this way:
<file>views/main.qml</file>
Similarly, the string in main.cpp would be qrc:/views/main.qml.
Once those changes are saved, you will see our empty main.qml file appear as a child of the qml.qrc file in the Projects pane. Double-click on that file to edit it, and we will finish off our project:
import QtQuick 2.9
import QtQuick.Window 2.3
Window {
visible: true
width: 1024
height: 768
title: qsTr("Scratchpad")
color: "#ffffff"
Text {
id: message
anchors.centerIn: parent
font.pixelSize: 44
text: qsTr("Hello Qt Scratchpad!")
color: "#008000"
}
}
We will cover QML in detail in Chapter 2, Project Structure, but in brief, this file represents the screen or view presented to the user when the application launches.
The import lines are similar to #include statements in C++, though rather than including a single header file, they import a whole module. In this case, we want the base QtQuick module to give us access to all the core QML types and also the QtQuick window module to give us access to the Window component. Modules are versioned and generally, you will want to use the latest version for the release of Qt you are using. The current version numbers can be found in the Qt documentation. Note that although you get code completion when entering the version numbers, the options presented sometimes don’t reflect the latest available versions.
As its name suggests, the Window element gives us a top-level window, inside which all of our other content will be rendered. We give it a size of 1024 x 765 pixels, a title of “scratchpad” and a background color of white represented as a hex RGB value.
Within that component (QML is a hierarchical markup language), we add a welcome message with the Text component. We center the text in the screen and set its font size and color, but other than that, we’re not concerned with fancy formatting or anything at this stage, so that’s as complicated as we’ll make it. Again, we’ll cover this in more detail later, so don’t worry if it seems a bit alien.
That’s it. To build and run our amazing new application, first select the Kit and Build configuration you want using the monitor icon at the bottom-left:

Next, right-click on the project name in the Projects pane and select Run qmake. When that has completed, Run the application using the green play icon:
