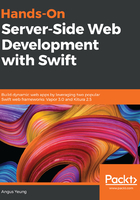
Adding more routes in Vapor
This section will show you how to add more routes in Vapor:
- Open the routes.swift file from Project Navigator on Xcode's left panel and add the following code to the line right before the closing brace at the bottom:
. . .
router.get("greet", String.parameter) { req -> String in // [1]
let guest = try req.parameters.next(String.self) // [2]
return "Hi \(guest), greetings! Thanks for visiting us." // [3]
}
. . .
- The preceding code does three things:
- Add a new route using greet as the first parameter from the path components and specifying the second parameter to be a string.
- Extract the guest string from the request's next item in its parameters list.
- Interpolate the guest string in the string returning to the client.
If you build and run your project again, you can see the following output by directing your web browser to http://localhost:8080/greet/Neil. Take note that the path is case-sensitive. For example, the N in Neil is capitalized:
- Now, you can add another route to show how your application will respond to different requests. Add the following code to the line right after the greet route but before the final ending brace:
. . .
router.get("student", String.parameter) { req -> String in
let studentName = try req.parameters.next(String.self) // [1]
let studentRecords = [ // [2]
"Peter" : 3.42,
"Thomas" : 2.98,
"Jane" : 3.91,
"Ryan" : 4.00,
"Kyle" : 4.00
]
if let gpa = studentRecords[studentName] { // [3]
return "The student \(studentName)'s GPA is \(gpa)" // [4]
} else {
return "The student's record can't be found!" // [5]
}
}
. . .
- The new route, student, will be added. Depending on the student's name, the logic handling the route will respond differently:
- Assign the next item on the request's parameters list to be the student name studentName
- Create a new array studentRecords that contains key-value pairs for the student's names and their GPA
- Unwrap the studentRecords using a student's name
- Format queryResponse with a student's name and their GPA
- Format queryResponse if the student's record can't be found
If you point your web browser to localhost:8080/student/Jane, you'll get an output text that looks like this:
Alternatively, you can run the curl command in a Terminal:
$ curl "http://localhost:8080/student/Jane"
The student Jane's GPA is 3.91
The student's name in client's request is used to check against the keys in an array with key-value pairs. You'll see in future chapters how we create controllers to implement logic in processing such query. The array of key-value pairs can be also replaced with a database for data recording and retrieval.