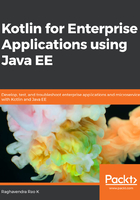
When clause
when is a pattern matching clause in Kotlin. This helps us to write elegant code when dealing with pattern matching, meaning we can avoid using a lot of if else statements.
Let's write a function that takes an input, the type of which is not known. Any is used to indicate this and a when block is used to handle different patterns.
Consider the code for 10_when.kts:
fun processInput(input: Any) {
when(input) {
10 -> println("It is 10")
98,99 -> println("It is 98 or 99")
in 101 .. 120 -> println("More than 100")
is String -> println("This is ${input} of length ${input.length}")
else -> println("Not known")
}
}
processInput(10)
processInput(98)
processInput(99)
processInput(102)
processInput("hey there")
processInput(Thread())
The output is as follows:
Alongside the pattern matching, we can also perform type checking using the when block:
is String -> println("This is ${input} of length ${input.length}")
Note that the argument input is of the Any type. After type checking, the input is automatically cast to String, and we can use the length property, which is defined in the String class. The Kotlin language does the auto-typecasting for us, so we don't have to do any explicit type casting.