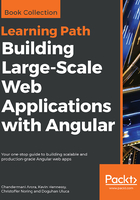
Fixing the saving of forms and validation messages
Open a new Workout page and directly click on the Save button. Nothing is saved as the form is invalid, but validations on individual form input do not show up at all. It now becomes difficult to know what elements have caused validation failure. The reason behind this behavior is pretty obvious. If we look at the error message binding for the name input element, it looks like this:
*ngIf="name.control?.hasError('required') && name.touched"
Remember that, earlier in the chapter, we explicitly disabled showing validation messages until the user has touched the input control. The same issue has come back to bite us and we need to fix it now.
We do not have a way to explicitly change the touched state of our controls to untouched. Instead, we will resort to a little trickery to get the job done. We'll introduce a new property called submitted. Add it at the top of the Workout class definition and set its initial value to false, like so:
submitted: boolean = false;
The variable will be set to true on the Save button click. Update the save implementation by adding the highlighted code:
save(formWorkout){ this.submitted = true; if (!formWorkout.valid) return; this._workoutBuilderService.save(); this.router.navigate(['/builder/workouts']); }
However, how does this help? Well, there is another part to this fix that requires us to change the error message for each of the controls we are validating. The expression now changes to:
*ngIf="name.control.hasError('required') && (name.touched || submitted)"
With this fix, the error message is shown when the control is touched or the form submit button is pressed (submitted is true). This expression fix now has to be applied to every validation message where a check appears.
If we now open the new Workout page and click on the Save button, we should see all validation messages on the input controls:
