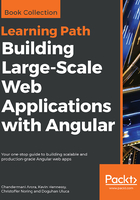
Angular CSS classes
Based on the model state, Angular adds some CSS classes to an input element. These include the following:
- ng-valid: This is used if the model is valid
- ng-invalid: This is used if the model is invalid
- ng-pristine: This is used if the model is pristine
- ng-dirty: This is used if the model is dirty
- ng-untouched: This is used when the input is never visited
- ng-touched: This is used when the input has focus
To verify it, go back to the workoutName input tag and add a template reference variable named spy inside the input tag:
<input type="text" name="workoutName" #name="ngModel" class="form-control" id="workout-name" placeholder="Enter workout name. Must be unique." [(ngModel)]="workout.name" required #spy>
Then, below the tag, add the following label:
<label>{{spy.className}}</label>
Reload the application and click on the New Workout link in the Workout Builder. Before touching anything on the screen, you will see the following displayed:

Add some content into the Name input box and tab away from it. The label changes to this:

What we are seeing here is Angular changing the CSS classes that apply to this control as the user interacts with it. You can also see these changes by inspecting the input element in the developer console.
These CSS class transitions are tremendously useful if we want to apply visual clues to the element depending on its state. For example, look at this snippet:
input.ng-invalid { border:2px solid red; }
This draws a red border around any input control that has invalid data.
As you add more validations to the Workout page, you can observe (in the developer console) how these classes are added and removed as the user interacts with the input element.
Now that we have an understanding of model states and how to use them, let's get back to our discussion of validations (before moving on, remove the variable name and label that you just added).