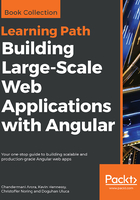
Implementing the Workout component continued...
Now that we have established the routing that takes us to the Workout component, let's turn to completing its implementation. So, copy the workout.component.ts file from the workout-builder/workout folder under trainer/src/app in checkpoint 4.5. (Also, copy workout-builder.module.ts from the workout-builder folder. We'll discuss the changes in this file a little later when we get to Angular forms.)
Open workout.component.ts and you'll see that we have added a constructor that injects ActivatedRoute and WorkoutBuilderService:
constructor( public route: ActivatedRoute, public workoutBuilderService:WorkoutBuilderService){ }
In addition, we have added the following ngOnInit method:
ngOnInit() {
this.sub = this.route.data
.subscribe(
(data: { workout: WorkoutPlan }) => {
this.workout = data.workout;
}
);
}
The method subscribes to the route and extracts the workout from the route.data. There is no need to check the workout exists because we have already done that in the WorkoutResolver.
We are subscribing to the route.data because as an ActivatedRoute, the route exposes its data as an Observable, which can change during the lifetime of the component. This gives us the ability to reuse the same component instance with different parameters, even though the OnInit life cycle event for that component is called only once. We'll cover Observables in detail in the next chapter.
In addition to this code, we have also added a series of methods to the Workout Component for adding, removing, and moving a workout. These methods all call corresponding methods on the WorkoutBuilderService and we will not review them in detail here. We've also added an array of durations for populating the duration drop-down list.
For now, this is enough for the component class implementation. Let's update the associated Workout template.