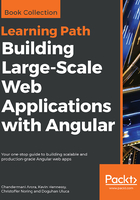
Route parameters
But before we get to building out the WorkoutComponentand its associated view, we need to touch briefly on the navigation that brings a user to the screen for that component. This component handles both creating and editing workout scenarios. The first job of the component is to load or create the workout that it needs to manipulate. We plan to use Angular's routing framework to pass the necessary data to the component, so that it will know whether it is editing an existing workout or creating a new one, and in the case of an existing workout, which component it should be editing.
How is this done? The WorkoutComponent is associated with two routes, namely /builder/workout/new and /builder/workout/:id. The difference in these two routes lies in what is at the end of these routes; in one case, it is /new, and in the other, /:id. These are called route parameters. The :id in the second route is a token for a route parameter. The router will convert the token to the ID for the workout component. As we saw earlier, this means that the URL that will be passed to the component in the case of 7 Minute Workout will be /builder/workout/7MinuteWorkout.
How do we know that this workout name is the right parameter for the ID? As you recall, when we set up the event for handling a click on the Workout tiles on the Workouts screen that takes us to the Workout screen, we designated the workout name as the parameter for the ID, like so:
onSelect(workout: WorkoutPlan) { this.router.navigate( ['./builder/workout', workout.name] ); }
Here, we are constructing the route using the programmatic interface for the router. The router.navigate method accepts an array. This is called the link parameters array. The first item in the array is the path of the route, and the second is a route parameter that specifies the ID of the workout. In this case, we set the id parameter to the workout name. We can also construct the same type of URL as part of a router link or simply enter it in the browser to get to the Workouts screen and edit a particular workout.
The other of the two routes ends with /new. Since this route does not have a token parameter, the router will simply pass the URL unmodified to the WorkoutComponent. The WorkoutComponent will then need to parse the incoming URL to identify that it should be creating a new component.