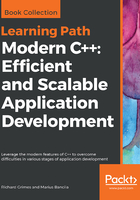
Defining class state
Your class can have built-in types as data members, or custom types. These data members can be declared in the class (and created when an instance of the class is constructed), or they can be pointers to objects created in the free store or references to objects created elsewhere. Bear in mind that if you have a pointer to an item created in the free store, you need to know whose responsibility it is to deallocate the memory that the pointer points to. If you have a reference (or pointer) to an object created on a stack frame somewhere, you need to make sure that the objects of your class do not live longer than that stack frame.
When you declare data members as public it means that external code can read and write to the data members.
You can decide that you would prefer to only give read-only access, in which case you can make the members private and provide read access through accessors:
class cartesian_vector
{
double x;
double y;
public:
double get_x() { return this->x; }
double get_y() { return this->y; }
// other methods
};
When you make the data members private it means that you cannot use the initializer list syntax to initialize an object, but we will address this later. You may decide to use an accessor to give write access to a data member and use this to check the value.
void cartesian_vector::set_x(double d)
{
if (d > -100 && d < 100) this->x = d;
}
This is for a type where the range of values must be between (but not including) -100 and 100.