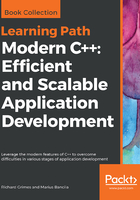
Using the this pointer
The methods in a class have a special calling convention, which in Visual C++ is called __thiscall. The reason is that every method in a class has a hidden parameter called this, which is a pointer of the class type to the current instance:
class cartesian_vector
{
public:
double x;
double y;
// other methods
double get_magnitude()
{
return std::sqrt((this->x * this->x) + (this->y * this->y));
}
};
Here, the get_magnitude method returns the length of the cartesian_vector object. The members of the object are accessed through the -> operator. As shown previously, the members of the class can be accessed without the this pointer, but it does make it explicit that the items are members of the class.
You could define a method on the cartesian_vector type that allows you to change its state:
class cartesian_vector
{
public:
double x;
double y;
reset(double x, double y) { this->x = x; this->y = y; }
// other methods
};
The parameters of the reset method have the same names as the data members of the class; however, since we use the this pointer the compiler knows that this is not ambiguous.
You can dereference the this pointer with the * operator to get access to the object. This is useful when a member function must return a reference to the current object (as some operators will, as we will see later) and you can do this by returning *this. A method in a class can also pass the this pointer to an external function, which means that it is passing the current object by reference through a typed pointer.