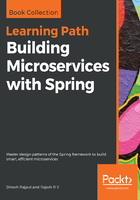
Best approach to configure the dependency injection pattern with Java
In the previous configuration example, I declared the transferService() bean method to construct an instance of the TransferServiceImpl class by using its arguments constructor. The bean methods, accountRepository() and transferRepository(), are passed as arguments of the constructor. But in an enterprise application, a lot of configuration files depend on the layers of the application architecture. Suppose the service layer and the infrastructure layer have their own configuration files. That means that the accountRepository() and transferRepository() methods may be in different configuration files, and the transferService() bean method may be in another configuration file. Passing bean methods into the constructor is not a good practice for configuration of the dependency injection pattern with Java. Let's see a different and the best approach to configuring the dependency injection:
package com.packt.patterninspring.chapter4.bankapp.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class AppConfig { @Bean public TransferService transferService(AccountRepository
accountRepository, TransferRepository transferRepository){ return new TransferServiceImpl(accountRepository,
transferRepository); } @Bean public AccountRepository accountRepository() { return new JdbcAccountRepository(); } @Bean public TransferRepository transferRepository() { return new JdbcTransferRepository(); } }
In the preceding code, the transferService() method asks for AccountRepository and TransferRepository as parameters. When Spring calls transferService() to create the TransferService bean, it autowires AccountRepository and TransferRepository into the configuration method. With this approach, the transferService() method can still inject AccountRepository and TransferRepository into the constructor of TransferServiceImpl without explicitly referring to the accountRepository() and transferRepository()@Bean methods.
Let's now take a look at dependency injection pattern with XML-based configuration.