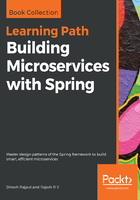
Declaring Spring beans into configuration class
To declare a bean in a Java-based configuration, you have to write a method for the desired type object creation in the configuration class, and annotate that method with @Bean. Let's see the following changes made in the AppConfig class to declare the beans:
package com.packt.patterninspring.chapter4.bankapp.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class AppConfig { @Bean public TransferService transferService(){ return new TransferServiceImpl(); } @Bean public AccountRepository accountRepository() { return new JdbcAccountRepository(); } @Bean public TransferRepository transferRepository() { return new JdbcTransferRepository(); } }
In the preceding configuration file, I declared three methods to create instances for TransferService, AccountRepository, and TransferRepository. These methods are annotated with the @Bean annotation to indicate that they are responsible for instantiating, configuring, and initializing a new object to be managed by the Spring IoC container. Each bean in the container has a unique bean ID; by default, a bean has an ID same as the @Bean annotated method name. In the preceding case, the beans will be named as transferService, accountRepository, and transferRepository. You can also override that default behavior by using the name attribute of the @Bean annotation as follows:
@Bean(name="service") public TransferService transferService(){ return new TransferServiceImpl(); }
Now "service" is the bean name of that bean TransferService.
Let's see how you can inject dependencies for the TransferService, AccountRepository, and TransferRepository beans in AppConfig.