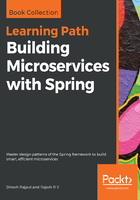
Wiring Beans using the Dependency Injection Pattern
In the previous chapter, you learned about the Gang of Four (GOF) design patterns with examples and use cases of each. Now, we will go into more detail about injecting beans and the configuration of dependencies in a Spring application, where you will see the various ways of configuring in a Spring application. This includes configuration with XML, Annotation, Java, and Mix.
Everyone loves movies, right? Well, if not movies, how about plays, or dramas, or theatre? Ever wondered what would happen if the different team members didn't speak to each other? By team I don't just mean the actors, but the sets team, make-up personnel, audio-visual guys, sound system guys, and so on. It is needless to say that every member has an important contribution towards the end product, and an immense amount of coordination is required between these teams.
A blockbuster movie is a product of hundreds of people working together toward a common goal. Similarly, great software is an application where several objects work together to meet some business target. As a team, every object must be aware of the other, and communicate with each other to get their jobs done.
In a banking system, the money transfer service must be aware of the account service, and the account service must be aware of the accounts repository, and so on. All these components work together to make the banking system workable. In Chapter 1, Getting Started with Framework 5.0 and Design Patterns, you saw the same banking example created with the traditional approach, that is, creating objects using construction and direct object initiation. This traditional approach leads to complicated code, is difficult to reuse and unit test, and is also highly coupled to one another.
But in Spring, objects have a responsibility to do their jobs without the need to find and create the other dependent objects that are required in their jobs. The Spring container takes the responsibility to find or create the other dependent objects, and to collaborate with their dependencies. In the previous example of the banking system, the transfer service depends on the account service, but it doesn't have to create the account service, so the dependency is created by the container, and is handed over to the dependent objects in the application.
In this chapter, we will discuss the behind-the-scenes story of the Spring-based application with reference to the dependency injection (DI) pattern, and how it works. By the end of this chapter, you will understand how the objects of your Spring-based application create associations between them, and how Spring wires these objects for a job done. You will also learn many ways to wire beans in Spring.
This chapter will cover the following topics:
- The dependency injection pattern
- Types of dependency injection patterns
- Resolving dependency using the Abstract Factory pattern
- Lookup-method injection pattern
- Configuring beans using the Factory pattern
- Configuring dependencies
- Common best practices for configuring dependencies in an application