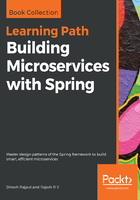
上QQ阅读APP看书,第一时间看更新
Sample implementation of the Builder design pattern
In the following code example, I am going to create an Account class that has AccountBuilder as an inner class. The AccountBuilder class has a method to create an instance of this class:
package com.packt.patterninspring.chapter2.builder.pattern; public class Account { private String accountName; private Long accountNumber; private String accountHolder; private double balance; private String type; private double interest; private Account(AccountBuilder accountBuilder) { super(); this.accountName = accountBuilder.accountName; this.accountNumber = accountBuilder.accountNumber; this.accountHolder = accountBuilder.accountHolder; this.balance = accountBuilder.balance; this.type = accountBuilder.type; this.interest = accountBuilder.interest; } //setters and getters public static class AccountBuilder { private final String accountName; private final Long accountNumber; private final String accountHolder; private double balance; private String type; private double interest; public AccountBuilder(String accountName,
String accountHolder, Long accountNumber) { this.accountName = accountName; this.accountHolder = accountHolder; this.accountNumber = accountNumber; } public AccountBuilder balance(double balance) { this.balance = balance; return this; } public AccountBuilder type(String type) { this.type = type; return this; } public AccountBuilder interest(double interest) { this.interest = interest; return this; } public Account build() { Account user = new Account(this); return user; } } public String toString() { return "Account [accountName=" + accountName + ",
accountNumber=" + accountNumber + ", accountHolder=" + accountHolder + ", balance=" + balance + ", type="
+ type + ", interest=" + interest + "]"; } }
AccountBuilderTest.java is a demo class that we will use to test the design pattern. Let's look at how to build an Account object by passing the initial information to the object:
package com.packt.patterninspring.chapter2.builder.pattern; public class AccountBuilderTest { public static void main(String[] args) { Account account = new Account.AccountBuilder("Saving
Account", "Dinesh Rajput", 1111l) .balance(38458.32) .interest(4.5) .type("SAVING") .build(); System.out.println(account); } }
You can test this file and see the output on the console:
