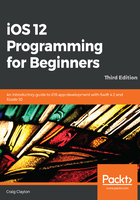
The repeat...while loop
The repeat...while loop is pretty similar to a while loop in that it continues to execute the set of statements until a condition becomes false. The main difference is that the repeat...while loop does not evaluate its bool condition until the end of the loop. Here is the basic syntax of a repeat...while loop:
repeat {
// statement
} <condition>
Let's write a repeat...while loop in Playgrounds and see how it works. Add the following to Playgrounds:
var x = 0
repeat {
x += 5
print("x: \(x)")
} while x < 100
print("repeat completed x: \(x)")

You will notice that our repeat...while loop executes first and increments x by 5, and after, as opposed to checking the condition like it did before, as with a while loop, it checks to see whether x is less than 100. This means that our repeat...while loop will continue until the condition hits 100. Here is where it gets interesting.
Let's add another repeat...while loop after the one we just created:
repeat {
x += 5
print("x: \(x)")
} while x < 100

Now, you can see that our repeat...while loop incremented to 105 instead of 100, like the previous repeat...while loop. This happens because the bool expression does not get evaluated until after it is incremented by 5. Knowing this behavior will help you to pick the correct loop for your situation.