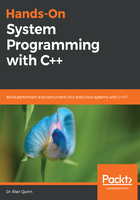
Error handling mechanism in C++
Error handling is another issue with C. The problem, at least until set jump exceptions were added, was that the only ways to get an error code from a function were as follows:
- Constrain the output of a function, so that certain output values from the function could be considered an error
- Get the function to return a structure, and then manually parse that structure
For example, consider the following code:
struct myoutput
{
int val;
int error_code;
}
struct myoutput myfunc(int val)
{
struct myoutput = {0};
if (val == 42) {
myoutput.error_code = -1;
}
myoutput.val = val;
return myoutput;
}
void
foo(void)
{
struct myoutput = myfunc(42);
if (myoutput.error_code == -1) {
printf("yikes\n");
return;
}
}
The preceding example provides a simple mechanism for outputting an error from a function without having to constrain the output of the function (for example, by assuming that -1 is always an error).
In C++, this can be implemented using the following C++17 logic:
std::pair<int, int>
myfunc(int val)
{
if (val == 42) {
return {0, -1};
}
return {val, 0};
}
void
foo(void)
{
if (auto [val, error_code] = myfunc(42); error_code == -1) {
printf("yikes\n");
return;
}
}
In the preceding example, we were able to remove the need for a dedicated structure by leveraging std::pair{}, and we were able to remove the need to work with std::pair{} by leveraging an initializer_list{} and C++17-structured bindings.
There is, however, an even easier method for handling errors without the need for checking the output of every function you execute, and that is to use exceptions. C provides exceptions through the set jump API, while C++ provides C++ exception support. Both of these will be discussed at length in Chapter 13, Error - Handling with Exceptions.