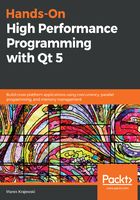
External functions
A similar problem poses itself to the compiler when we are calling a function that isn't in the current compilation unit and is hence somewhere the compiler cannot peep into. In such a case, a compiler has to assume the worst. Let's discuss it using another summation example:
int mutatingFunc(int value);
int sum(const vector<int>& v)
{
int sum = 0;
for(std::size_t i = 0; i < v.size(); ++i)
{
sum += mutatingFunc(v[i]);
}
return sum;
}
So, where's the problem? The compiler cannot assume that the mutatingFunc() didn't change the input vector. How come? Well, if the vector is somehow globally visible to the function, it can be theoretically mutated as well! Maybe you have heard about the concept of the pure functions used in functional languages such as Haskell. These are functions that only use their input parameter to calculate the result and don't touch anything else. C++ doesn't have a pure keyword for now, so we have to fall back on compiler extensions, such as GCC attributes, to denote a function's purity:
int mutatingFunc2(int value) __attribute__((pure));
So, as I think that we have gained enough knowledge on compilers' inner workings by now, we are in a position to answer the following question: since the compiler helps us so much, what can we do, in turn, to help the compiler?