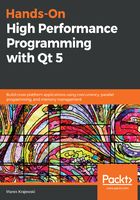
Expression templates
Expression templates were an optimization technique introduced by Ted Veldhuisen in his implementation of the Blitz++ numerics library. It may be too complicated to explain them in an intermediate-level book, but the general idea is to build up a nested type definition containing all of the information about an expression, and then, after all of the information has been gathered, to create the final code.
One example could be concatenating strings: instead of naively creating temporary strings after each concatenation (as shown in the previous section on temporaries), we could first collect all of the string information, then allocate a buffer big enough to store all of the strings to be concatenated and fill it in the last phase.
It sounds like magic—can we do that all at compile time? Yes, with enough ugly template code we can. The idea is to use operator overloading and somehow poison the expression so that the expression template overload will be chosen. Look at the following code to get the general idea:
template <typename L, typename R>
StrgSumExpr { ... };
template <typename L, typename R>
StrgSumExpr<L, R> operator+(const L& lhs, const R& rhs)
{
return StrgSumExpr<L, R>(lhs, rhs);
}
class String
{
...
template <typename L, typename R>
String& operator=(const StrgSumExpr<L, R>& expression)
{
reserve(expr.size());
auto count = expression.count();
// concatenate in reverse order
for(size_t i = 0; i < count; ++i)
{
append(expression[count - 1 - i]);
}
return *this;
}
};
Does it help? Well, it is a technique that avoids the creation of temporary objects while still supporting clear code, hence it is a workaround for a C++ limitation. So, the answer is: yes, it can help in some cases.