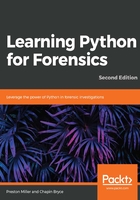
Understanding the get_address() function
This is an integral, though a potentially error-prone, component of our script because it relies on the user correctly supplying data. The code itself is just a simple data request. However, when working with user supplied arguments, it isn't safe to assume that the user gave the script the correct data. Considering the length and somewhat random-looking sequence of a Bitcoin address, it's entirely possible that the user might supply an incorrect address. We'll catch any instance of URLError from the urllib.error module to handle a malformed input. URLError isn't part of the built-in exceptions we've talked about before and is a custom exception defined by the urrlib module:
053 def get_address(address):
054 """
055 The get_address function uses the blockchain.info Data API
056 to pull pull down account information and transactions for
057 address of interest
058 :param address: The Bitcoin Address to lookup
059 :return: The response of the url request
060 """
On line 62, we insert the user-supplied address into the blockchain.info API call using the string format() method. Then, we try to return the data requested using the urllib.request.urlopen() function. If the user supplies an invalid address or if the user doesn't have an internet connection, URLError will be caught. Once the error has been caught, we notify the user and exit the script, calling sys.exit(1) on line 67:
061 url = 'https://blockchain.info/address/{}?format=json'
062 formatted_url = url.format(address)
063 try:
064 return urllib.request.urlopen(formatted_url)
065 except urllib.error.URLError:
066 print('Received URL Error for {}'.format(formatted_url))
067 sys.exit(1)