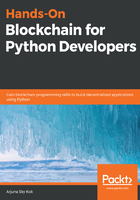
Blockchain technology
Most people know Bitcoin exists because of blockchain. But what is blockchain? It is an append-only database that consists of blocks that are linked by hashing. Here, each block contains many transactions of transferring value (but could be other things) between participants secured by cryptography; a consensus between many nodes that hold an identical database decides on which new block is to be appended next.
You don't have to understand the definition at this point; those are a lot of words to chew on! First, I'll explain blockchain to you so that you can adjust to this new knowledge as we move through this book.
Going back to the definition of blockchain, we can summarize the definition as an append-only database. Once you put something into the database, it cannot be changed; there is no Undo. We'll talk about the ramifications of this feature in Chapter 2, Smart Contract Fundamentals. This definition entails many things and opens up a whole new world.
So, what can you put into this append-only database? It depends on the cryptocurrency. For Bitcoin, you can store the transactions of transferring value. For example, Nelson sends one Bitcoin to Dian. However, we accumulate many transactions into one block before appending them to the database. For Ethereum, the things that you can put into the append-only database are richer. This not only includes the transaction of transferring value—it could also be a change of state. What I mean by state here is really general. For example, a queue for buying a ticket for a show can have a state. This state can be empty or full. Similarly to Bitcoin, in Ethereum, you gather all the transactions before appending them together in this append-only database.
To make it clearer, we put all these transactions into the block before appending them to the append-only database. Aside from the list of transactions, we store other things in this block, such as the time when we append the block into the append-only database, the target's difficulty (don't worry if you don't know about this), and the parent's hash (I'll explain this shortly), among many other things.
Now that you understand the block element of the blockchain, let's look at the chain element. As previously explained, aside from the list of transactions, we also put the parent's hash in the block. But for now, let's just use a simple ID to indicate the parent instead of using a hash. Parent id is just the previous block id. Here, think of the stack. In the beginning, there is no block. Instead, we put Block A, which has three transactions: Transaction 1, Transaction 2, and Transaction 3. Since Block A is the first block, it has no parent. We then apply Block B to Block A, which consists of two transactions: Transaction 4 and Transaction 5. Block B is not the first one in this blockchain. Consequently, we set the parent section in Block B as the Block A id because Block A is the parent of Block B. Then, we put Block C in the blockchain, which has two transactions: Transaction 6 and Transaction 7.
The parent section in Block C would be the Block B id, and so on. To simplify things, we increment the id from 0 by 1 for every new block:

Let's implement a database to record the history of what people like and hate. This means that when you said you like cats at one point in history, you won't be able to change that history. You may add new history when you change your mind (for example, if you then hate cats), but that won't change the fact that you liked them in the past. So, we can see that in the past you liked cats, but now you hate them. We want to make this database full of integrity and secure against cheating. Take a look at the following code block:
class Block:
id = None
history = None
parent_id = None
block_A = Block()
block_A.id = 1
block_A.history = 'Nelson likes cat'
block_B = Block()
block_B.id = 2
block_B.history = 'Marie likes dog'
block_B.parent_id = block_A.id
block_C = Block()
block_C.id = 3
block_C.history = 'Sky hates dog'
block_C.parent_id = block_B.id
If you studied computer science, you will recognize this data structure, which is called a linked list. Now, there is a problem. Say Marie hates Nelson and wants to paint Nelson in a negative light. Marie can do this by changing the history of block A:
block_A.history = 'Nelson hates cat'
This is unfair to Nelson, who is a big fan of cats. So, we need to add a way in which only Nelson can write the history of his own preferences. The way to do this is by using a private key and a public key.