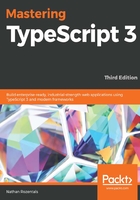
Building a simple HTML application
Now that we have configured WebStorm to compile our Typescript files, let's create a simple TypeScript class and use it to modify the innerText property of an HTML div. While you are typing, you will notice WebStorm's autocompletion or Intellisense feature helping you with available keywords, parameters, and naming conventions, among others. This is one of the most powerful features of WebStorm, and is similar to the enhanced Intellisense seen in Visual Studio. To see a list of TypeScript compilation errors, we can open the TypeScript output window by navigating to View | Tool Windows | TypeScript. As we type code into this file, WebStorm will automatically compile our file ( without needing to save it), and report any errors in the TypeScript tool window. Go ahead and type the following TypeScript code, during which you will get a good feeling of WebStorm's available autocompletion, and the error-reporting capabilities:
class MyClass { public render(divId: string, text: string) { var el: HTMLElement | null = document.getElementById(divId); if (el) { el.innerText = text; } } } window.onload = () => { var myClass = new MyClass(); myClass.render("content", "Hello World"); }
This code is similar to the sample we used for Visual Studio 2017.
If you have any errors in your TypeScript file, these will automatically show up in the output window, giving you instant feedback while you type.
With this TypeScript file created, we can now include it in our index.html file and try some debugging.
Open the index.html file, and add a script tag to include the app.js JavaScript file, along with a div with an id of content. Just as we saw with TypeScript editing, you will find that WebStorm has powerful Intellisense features when editing HTML as well:
<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> <script src="app.js"></script> </head> <body> <div id="content"></div> </body> </html>
Again, this HTML is the same as we used earlier in the Visual Studio example.