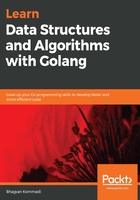
The AddToHead method
The AddToHead method adds the node to the start of the linked list. The AddToHead method of the LinkedList class has a parameter integer property. The property is used to initialize the node. A new node is instantiated and its property is set to the property parameter that's passed. The nextNode points to the current headNode of linkedList, and headNode is set to the pointer of the new node that's created, as shown in the following code:
//AddToHead method of LinkedList class
func (linkedList *LinkedList) AddToHead(property int) {
var node = Node{}
node.property = property
if node.nextNode != nil {
node.nextNode = linkedList.headNode
}
linkedList.headNode = &node
}
When the node with the 1 property is added to the head, adding the 1 property to the head of linkedList sets headNode to currentNode with a value of 1, as you can see in the following screenshot:

Let's execute this command using the main method. Here, we have created an instance of a LinkedList class and added the 1 and 3 integer properties to the head of this instance. The linked list's headNode property is printed after adding the elements, as follows:
// main method
func main() {
var linkedList LinkedList
linkedList = LinkedList{}
linkedList.AddToHead(1)
linkedList.AddToHead(3)
fmt.Println(linkedList.headNode.property)
}
Run the following commands to execute the linked_list.go file:
go run linked_list.go
After executing the preceding command, we get the following output:

Let's take a look at the IterateList method in the next section.