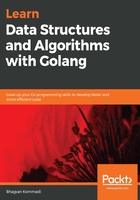
CRUD web forms
In this section, we will explain web forms using basic examples, showing you how to perform various actions.
To start a basic HTML page with the Go net/http package, the web forms example is as follows (webforms.go). This has a welcome greeting in main.html:
//main package has examples shown
// in Hands-On Data Structures and algorithms with Go book
package main
// importing fmt, database/sql, net/http, text/template package
import (
"net/http"
"text/template"
"log")
// Home method renders the main.html
func Home(writer http.ResponseWriter, reader *http.Request) {
var template_html *template.Template
template_html = template.Must(template.ParseFiles("main.html"))
template_html.Execute(writer,nil)
}
// main method
func main() {
log.Println("Server started on: http://localhost:8000")
http.HandleFunc("/", Home)
http.ListenAndServe(":8000", nil)
}
The code for main.html is as follows:
<html>
<body>
<p> Welcome to Web Forms</p>
</body>
</html>
Run the following commands:
go run webforms.go
The following screenshot displays the output:

The web browser output is shown in the following screenshot:

The CRM application is built with web forms as an example to demonstrate CRUD operations. We can use the database operations we built in the previous section. In the following code, the crm database operations are presented. The crm database operations consist of CRUD methods such as CREATE, READ, UPDATE, and DELETE customer operations. The GetConnection method retrieves the database connection for performing the database operations (crm_database_operations.go):
//main package has examples shown
// in Hands-On Data Structures and algorithms with Go book
package main
// importing fmt,database/sql, net/http, text/template package
import (
"database/sql"
_ "github.com/go-sql-driver/mysql"
)
// Customer Class
type Customer struct {
CustomerId int
CustomerName string
SSN string
}
// GetConnection method which returns sql.DB
func GetConnection() (database *sql.DB) {
databaseDriver := "mysql"
databaseUser := "newuser"
databasePass := "newuser"
databaseName := “crm"
database, error := sql.Open(databaseDriver, databaseUser+”:"+databasePass+"@/"+databaseName)
if error != nil {
panic(error.Error())
}
return database
}
As shown in the following code, the GetCustomerById method takes the customerId parameter to look up in the customer database. The GetCustomerById method returns the customer object:
//GetCustomerById with parameter customerId returns Customer
func GetCustomerById(customerId int) Customer {
var database *sql.DB
database = GetConnection()
var error error
var rows *sql.Rows
rows, error = database.Query("SELECT * FROM Customer WHERE CustomerId=?",customerId)
if error != nil {
panic(error.Error())
}
var customer Customer
customer = Customer{}
for rows.Next() {
var customerId int
var customerName string
var SSN string
error = rows.Scan(&customerId, &customerName, &SSN)
if error != nil {
panic(error.Error())
}
customer.CustomerId = customerId
customer.CustomerName = customerName
customer.SSN = SSN
}
Now that we have covered CRUD web forms, let's move on to defer and panic in the next section.