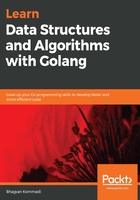
上QQ阅读APP看书,第一时间看更新
Slice function
Slices are passed by referring to functions. Big slices can be passed to functions without impacting performance. Passing a slice as a reference to a function is demonstrated in the code as follows (slices.go):
//twiceValue method given slice of int type
func twiceValue(slice []int) {
var i int
var value int
for i, value = range slice {
slice[i] = 2*value
}
}
// main method
func main() {
var slice = []int{1,3,5,6}
twiceValue(slice)
var i int
for i=0; i< len(slice); i++ {
fmt.Println(“new slice value”, slice[i])
}
}
Run the following command:
go run slices.go
The following screenshot displays the output:

Now that we know what slices are, let's move on to two-dimensional slices in the next section.