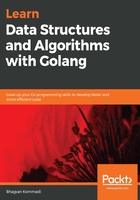
Logarithmic complexity
An algorithm is of logarithmic complexity if the processing time is proportional to the logarithm of the input elements. The logarithm base is typically 2. The following tree is a binary tree with LeftNode and RightNode. The insert operation is of O(log n) complexity, where n is the number of nodes.
Logarithmic complexity is presented as follows:
//main package has examples shown
// in Hands-On Data Structures and algorithms with Go book
package main
// importing fmt package
import (
"fmt"
)
// Tree struct
type Tree struct {
LeftNode *Tree
Value int
RightNode *Tree
}
As shown in the following code, the Tree class has the insert method, which inserts the element given m is the integer element:
// Tree insert method for inserting at m position
func (tree *Tree) insert( m int) {
if tree != nil {
if tree.LeftNode == nil {
tree.LeftNode = &Tree{nil,m,nil}
} else {
if tree.RightNode == nil {
tree.RightNode = &Tree{nil,m,nil}
} else {
if tree.LeftNode != nil {
tree.LeftNode.insert(m)
} else {
tree.RightNode.insert(m)
}
}
}
} else {
tree = &Tree{nil,m,nil}
}
}
//print method for printing a Tree
func print(tree *Tree) {
if tree != nil {
fmt.Println(" Value",tree.Value)
fmt.Printf("Tree Node Left")
print(tree.LeftNode)
fmt.Printf("Tree Node Right")
print(tree.RightNode)
} else {
fmt.Printf("Nil\n")
}
}
The main method calls the insert method on tree to insert the 1, 3, 5, and 7 elements, as shown in the following code:
// main method
func main() {
var tree *Tree = &Tree{nil,1,nil}
print(tree)
tree.insert(3)
print(tree)
tree.insert(5)
print(tree)
tree.LeftNode.insert(7)
print(tree)
}
Run the following commands:
go run tree.go
The following screenshot displays the output:

Now that we know about the complexities in algorithms and analyzing their performance, let's take a look at brute force algorithms in the next section.