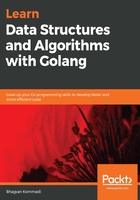
Lists
A list is a sequence of elements. Each element can be connected to another with a link in a forward or backward direction. The element can have other payload properties. This data structure is a basic type of container. Lists have a variable length and developer can remove or add elements more easily than an array. Data items within a list need not be contiguous in memory or on disk. Linked lists were proposed by Allen Newell, Cliff Shaw, and Herbert A. Simon at RAND Corporation.
To get started, a list can be used in Go, as shown in the following example; elements are added through the PushBack method on the list, which is in the container/list package:
//main package has examples shown
// in Hands-On Data Structures and algorithms with Go book
package main
// importing fmt and container list packages
import (
"fmt"
"container/list")
// main method
func main() {
var intList list.List
intList.PushBack(11)
intList.PushBack(23)
intList.PushBack(34)
for element := intList.Front(); element != nil; element=element.Next() {
fmt.Println(element.Value.(int))
}
}
The list is iterated through the for loop, and the element's value is accessed through the Value method.
Run the following commands:
go run list.go
The following screenshot displays the output:

Let's take a look at Tuples in the next section.