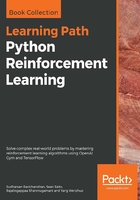
Adding scope
Scoping is used to reduce complexity and helps us to better understand the model by grouping the related nodes together. For instance, in the previous example, we can break down our graph into two different groups called computation and result. If you look at the previous example, you can see that nodes a to e perform the computation and node g calculates the result. So we can group them separately using the scope for easy understanding. Scoping can be created using the tf.name_scope() function.
Let's use the tf.name_scope() function using Computation:
with tf.name_scope("Computation"):
a = tf.constant(5)
b = tf.constant(4)
c = tf.multiply(a,b)
d = tf.constant(2)
e = tf.constant(3)
f = tf.multiply(d,e)
Let's use the tf.name_scope() function using Result:
with tf.name_scope("Result"):
g = tf.add(c,f)
Look at the Computation scope; we can further break down into separate parts for even more understanding. We can create a scope as Part 1, which has nodes a to c, and a scope as Part 2, which has nodes d to e, as part 1 and 2 are independent of each other:
with tf.name_scope("Computation"):
with tf.name_scope("Part1"):
a = tf.constant(5)
b = tf.constant(4)
c = tf.multiply(a,b)
with tf.name_scope("Part2"):
d = tf.constant(2)
e = tf.constant(3)
f = tf.multiply(d,e)
Scoping can be better understood by visualizing them in the TensorBoard. The complete code is as follows:
import tensorflow as tf
with tf.name_scope("Computation"):
with tf.name_scope("Part1"):
a = tf.constant(5)
b = tf.constant(4)
c = tf.multiply(a,b)
with tf.name_scope("Part2"):
d = tf.constant(2)
e = tf.constant(3)
f = tf.multiply(d,e)
with tf.name_scope("Result"):
g = tf.add(c,f)
with tf.Session() as sess:
writer = tf.summary.FileWriter("output", sess.graph)
print(sess.run(g))
writer.close()
If you look at the following diagram, you can easily understand how scope helps us to reduce complexity in understanding by grouping the similar nodes together. Scoping is widely used while working on a complex project to better understand the functionality and dependencies of nodes:
