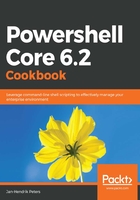
上QQ阅读APP看书,第一时间看更新
How to do it...
Install and start PowerShell Core and execute the following steps:
- Try the following cmdlets and observe the result:
# This command will return both an error and one output object
Get-Item -Path $home,'doesnotexist'
# This command usually returns nothing. The Verbose parameter however enables another stream.
Remove-Item -Path $(New-TemporaryFile) -Verbose
- The Verbose parameter was actually part of the common parameters. Run the next lines of code and observe what happens now:
Get-Item -Path $home,'doesnotexist' -ErrorAction SilentlyContinue -OutVariable file -ErrorVariable itemError
$file.FullName # Yes, it's your file
$itemError.Exception.GetType().FullName # This sure looks like your error
- Like the Verbose and Debug streams, the information stream isn't visible by default. Try executing the following code to see the versatility of the information stream:
function Get-AllTheInfo
{
[CmdletBinding()]param()
Write-Information -MessageData $(Get-Date) -Tags Dev,CIPipelineBuild
if ($(Get-Date).DayOfWeek -notin 'Saturday','Sunday')
{
Write-Information -MessageData "Get to work, you slacker!" -Tags Encouragement,Automation
}
}
# Like the Verbose and Debug streams, Information isn't visible by default
# Working with the information is much improved by cmdlets that actually process the tags
Get-AllTheInfo -InformationVariable infos
Get-AllTheInfo -InformationAction Continue
# Information can be filtered and processed, allowing more sophisticated messages in your scripts
$infos | Where-Object -Property Tags -contains 'CIPipelineBuild'
- Streams can also be controlled globally through the Preference variables as the next sample shows:
$ErrorActionPreference = 'SilentlyContinue'
$VerbosePreference = 'Continue'
Import-Module -Name Microsoft.PowerShell.Management -Force
Get-Item -Path '/somewhere/over/the/rainbow'
- Additional parameters exist for the purpose of risk mitigation. You might already know them as WhatIf and Confirm. Take a look at the following example:
Remove-Item -Path (New-TemporaryFile) -Confirm
Remove-Item -Path (New-TemporaryFile) -WhatIf
# WhatIf and Confirm are governed by automatic variables as well
$WhatIfPreference = $true
New-TemporaryFile
$WhatIfPreference = $false # Reset to default
$ConfirmPreference = 'Low' # None,Low,Medium,High
New-TemporaryFile
- As a cmdlet developer, try to use the Write cmdlets (apart from Write-Host) to write to different streams:
Write-Warning -Message 'A warning looks like this'
Write-Error -Message 'While an error looks like this'
Write-Verbose -Message 'Verbose, Debug and Information are hidden by default'