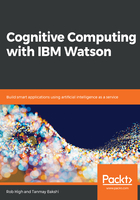
Programming your conversation application
So, now we're ready to start developing an application to make use of your trained Watson conversation. For this, we're going to use Python, and we're less concerned with the proper structuring of the application as a whole than with the structuring of your calls to the Watson Assistant services. However, before we begin, we need to get the Python SDK for Watson. If you don't already have Python installed, go to https://www.python.org/.
Once you have Python installed, you should be able to use the PIP tool to download and install the Watson SDK by issuing the following command in a terminal window:
pip install --upgrade ibm-watson
There are occasions where you may run into permission errors when you issue that command on Mac or Linux. If you do, type the following command:
sudo -H pip install --ignore-installed six ibm-watson
More information about the software developer kit (SDK) can be found at https://github.com/watson-developer-cloud/python-sdk. The Watson developer SDKs (for a variety of programming languages) make it convenient and very easy to invoke Watson services from your laptop, smartphone, a server, another Cloud—virtually anywhere you want to run your application.
You will also need to install other dependencies as described in: https://github.com/watson-developer-cloud/python-sdk#dependencies.
The first thing you will need to do in your program is to import the ibm_watson library, like this:
import ibm_watson
Of course, you may want to import other libraries that will be useful to your application, such as the json library.
You will then create a session with the Watson Assistant service you created earlier in this chapter. The code for that looks like this:
[Chp2-Program-1.py]
assistant = ibm_watson.AssistantV1(
iam_apikey='PrLJLVykZG4V-FQrADLiZG91oOKcJN0UZWEUAo0HxW8Q',
version='2018-09-20')
You will get the apikey value from the Credentials page for the service. You can get to them by pressing the Deploy (looks like a circular arrow) button to the left of the conversation editor:

This will bring up the Deploy page. You can then press the Credentials tab:

The Username and Password are listed on the page, like this:

Just copy and paste the values from the screen into your program.
The version is provided to you as part of the API specification, which you can find at https://www.ibm.com/watson/developercloud/assistant/api/v1/python.html?python#introduction.
Once you have the session established, you can go on to call the Watson Assistant service, like this:
[Chp2-Program-2.py]
response = assistant.message(
workspace_id='3e86c7a1-b071-4e6a-ada2-a8ac616e6aa6'
).get_result()
workspace_id is the identity of the specific conversation workspace that you have created. Again, you can do that from the Deploy Credentials page, as seen in the following screenshot:

As before, just cut and paste the Workspace ID value into your application.
We should also take a moment to explain what else is going on in this piece of code. We're making a call to the message() function on the AssistantV1 class instance that we created when we opened a session to the Watson Assistant. We're passing in the workspace ID that we want to operate on, and we're getting back a response that we retrieve with the get_result() function.
The response is a JSON structure returned by the service and we can use standard Python JSON operations to parse.
If you want to visually examine the contents of the returned response, you use the json.dumps() function within a print() statement, as follows. This will print out the JSON contents in a readable form:
print(json.dumps(response, indent=2))
We can make this call to Watson without the benefit of any input from the user. To do this the first time, typically, we prompt Watson for whatever greeting was established to open the conversation.
Remember the Welcome node in your dialog that we created earlier in this chapter? It looked something like the following:

The Welcome node describes the opening text of your dialog. Having retrieved it from Watson, you can now present it to the user to prompt them for input, and subsequently request their input. Having got their input utterance, you can then submit that back to Watson for further processing—perhaps in a loop that goes back and forth between the following:
- Requesting input from the user
- Processing that with Waston
- Presenting the response from Watson back to the user
This will continue until the conversation terminates in a graceful manner.
The call to Watson within this loop might look more like the following:
[Chp2-Program-2.py]
response = assistant.message(
workspace_id='3e86c7a1-b071-4e6a-ada2-a8ac616e6aa6',
input={'text': utterance}, context=response.get("context")
).get_result()
As before, we are supplying the workspace_id, but this time we're supplying two other parameters: the user's utterance, which is supplied as a JavaScript object notation (JSON) input string, and the context retrieved from the prior call to Watson—the latter is needed to retain some fluidity between each interaction with Watson.
A more complete example application might look like the following:

We call this function BankBot(). Let's examine it in more detail:
- On line 7 we are creating the session to Watson Assistant.
- On line 12 we call the assistant to get its opening statement.
- We go into a loop on line 17.
- On line 20 we are getting the text that Watson would have us present to the user.
- Keep in mind that Watson may have us present multiple lines of text, so, on line 23, we are printing however many lines of text Watson has given us.
- On line 27, we prompt the user for their utterance, which we pass back into Watson on line 31, along with the context from the prior invocation of Watson.
- On line 38, we check whether Watson has decided to terminate the conversation (generally due to something the user said)—in this case by looking for the General_Ending intent.
- If this is the end of the conversation, we check whether there are any last things Watson would have us tell the user—usually a salutation of some sort—and then we break out of the loop on line 47.
This may result in a conversation that looks something like this:

More typically, your application will get involved in the conversation by acting on various intents, such as performing the actual transfer of funds or leveraging an existing backend transaction system. That logic might typically be inserted at the same point where other checks, such as ending the conversation, are being performed.