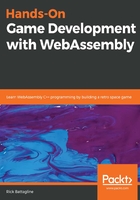
Defining the shaders
I walked through everything that's being done by the shaders in the previous section. You may want to go through that section again as a refresher. The next part of the code defines the vertex shader code and the fragment shader code in multiline JavaScript strings. Here is the vertex shader code:
var vertex_shader_code = `
precision mediump float;
attribute vec4 a_position;
attribute vec2 a_texcoord;
varying vec2 v_texcoord;
uniform vec4 u_translate;
void main() {
gl_Position = u_translate + a_position;
v_texcoord = a_texcoord;
}
`;
The fragment shader code is as follows:
var fragment_shader_code = `
precision mediump float;
varying vec2 v_texcoord;
uniform sampler2D u_texture;
void main() {
gl_FragColor = texture2D(u_texture, v_texcoord);
}
`;
Let's take a look at the attribute in the vertex shader code:
attribute vec4 a_position;
attribute vec2 a_texcoord;
Those two attributes will be passed in from the data in Float32Array. One of the neat tricks in WebGL is that if you are not using all four position variables (x,y,z,w), you can pass in the two you are using (x,y) and the GPU will know how to use appropriate values in the other two positions. These shaders will require passing in two attributes:
attribute vec4 a_position;
attribute vec2 a_texcoord;
Once again, we will be doing this using buffers and Float32Array. We will also need to pass in two uniform variables. The u_translate variable will be used by the vertex shader to translate the position of the sprite, and u_texture is a texture buffer that will be used by the fragment shader. These shaders are almost as simple as they get. Many tutorials start you out without a texture and just hardcode the color output of the fragment shader, like this:
gl_FragColor = vec4(1.0, 0.0, 0.0, 1.0);
Making this change would cause the fragment shader to always output a red color, so please don't make this change. The only things I can think of that could have made this tutorial simpler are not loading the texture and rendering a solid color, and not allowing the geometry to be moved.