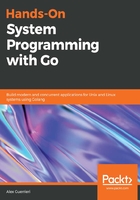
上QQ阅读APP看书,第一时间看更新
Looping
The for statement is the only looping statement in Go. This requires that you specify three expressions, separated by ;:
- A short declaration or an assignment to an existing variable
- A condition to verify before each iteration
- An operation to execute at the end of the iteration
All of these statements can be optional, and the absence of a condition means that it is always true. A break statement interrupts the loop's execution, while continue skips the current iteration and proceeds with the next one:
for { // infinite loop
if condition {
break // exit the loop
}
}
for i < 0 { // loop with condition
if condition {
continue // skip current iteration and execute next
}
}
for i:=0; i < 10; i++ { // loop with declaration, condition and operation
}
When a combination of switch and for are nested, the continue and break statements refer to the inner flow control statement.
An outer loop or condition can be labelled using a name: expression, whereas the name is its identifier, and both loop and continue can be followed by the name in order to specify where to intervene, as shown in the following code:
label:
for i := a; i<a+2; i++ {
switch i%3 {
case 0:
fmt.Println("divisible by 3")
break label // this break the outer for loop
default:
fmt.Println("not divisible by 3")
}
}