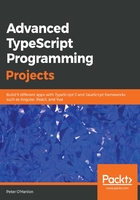
Validating the phone number
The phone number validation is going to be broken down into two parts. First, we validate that there is an entry for the phone number. Then, we validate to ensure that it is in the correct format using a regular expression. Before we analyze the regular expression, let's see what this validation class looks like:
export class PhoneValidation implements IValidation {
private readonly regexValidator : RegularExpressionValidator = new RegularExpressionValidator(`^(?:\\((?:[0-9]{3})\\)|(?:[0-9]{3}))[-. ]?(?:[0-9]{3})[-. ]?(?:[0-9]{4})$`);
private readonly minLengthValidator : MinLengthValidator = new MinLengthValidator(1);
public Validate(state : IPersonState, errors : string[]) : void {
if (!this.minLengthValidator.IsValid(state.PhoneNumber)) {
errors.push("You must enter a phone number")
} else if (!this.regexValidator.IsValid(state.PhoneNumber)) {
errors.push("The phone number format is invalid");
}
}
}
The regular expression initially looks more complicated than the zip code validation; however, once we break it down, we will see that it has lots of familiar elements. It uses ^ to capture from the start of the line, $ to capture right to the end, and ?: to create non-capture groups. We also see that we have set number matches such as [0-9]{3} to represent three numbers. If we break this down section by section, we will see that this really is a straightforward piece of validation.
The first part of our phone number either takes the format of (555) or 555 optionally followed by a hyphen, period, or space. At first glance, (?:\\((?:[0-9]{3})\\)|(?:[0-9]{3}))[-. ]? is the most intimidating part of the expression. As we know, the first part either has to be something such as (555) or 555; that means that we have either this expression or this expression test. We have already seen that ( and ) mean something special to the regular expression engine so we must have some mechanism available to us to say that we are looking at the actual brackets rather than the expression that the brackets represent. That is what the \\ part means in the expression.
When we want a regular expression to say a value must be this or that, we group the expression and then use | to break it apart. Looking at our expression there, we see that we are looking for the (nnn) part first and, if that is not matched, we look at the nnn part instead.
We also said that this value could be followed by a hyphen, period, or space. We use [-. ] to match a single character from that list. To make this test optional, we put ? at the end.
With this knowledge, we see that the next part of the regular expression, (?:[0-9]{3})[-. ]?, is looking for three numbers optionally followed by a hyphen, period, or space. The final part, (?:[0-9]{4}), states that the number must end in four digits. We now know that we can match numbers such as (555) 123-4567, 123.456.7890, and (555) 543 9876.