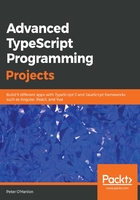
Simplifying type declarations with type aliases
Something that goes hand in hand with intersection types and union types are type aliases. Rather than cluttering our code with references to string | number | null, TypeScript gives us the ability to create a handy alias that is expanded out by the compiler into the relevant code.
Suppose that we want to create a type alias that represents the union type of string | number, then we can create an alias that looks as follows:
type StringOrNumber = string | number;
If we revisit our range validation sample, we can change the signature of our function to use this alias, as follows:
class UnionRangeValidationWithTypeAlias extends RangeValidationBase {
IsInRange(value : StringOrNumber) : boolean {
if (typeof value === "number") {
return this.RangeCheck(value);
}
return this.RangeCheck(this.GetNumber(value));
}
}
The important thing to notice in this code is that we don't really create any new types here. The type alias is just a syntactic trick that we can use to make our code more readable and, more importantly, help us to create code that is more consistent when we are working in larger teams.
We can combine type aliases with types to create more complex type aliases as well. If we wanted to add null support to the previous type alias, we could add this type:
type NullableStringOrNumber = StringOrNumber | null;
As the compiler still sees the underlying type and uses that, we can use the following syntax to call our IsInRange method:
let total : string | number = 10;
if (new UnionRangeValidationWithTypeAlias(0,100).IsInRange(total)) {
console.log(`This value is in range`);
}
Obviously, this doesn't give us very consistent-looking code, so we can change string | number to StringOrNumber.