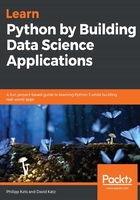
Comprehensions
Comprehensions are a nice and expressive way to work with data structures. Let's start with a simple example:
{el**2 for el in range(3)}
>>> {0, 1, 4}
Here, the curly brackets define our result. We use range to create the initial iterable, and then loop over its values, computing the square value of each. This is not a real loop, though. List comprehensions are actually faster than loops and even map, as there are no lambdas, and thus, no additional costs for stack lookups:
>>> %%timeit
... s = set()
... for el in range(10):
... s.add(el**2)
3.35 µs ± 134 ns per loop (mean ± std. dev. of 7 runs, 100000 loops each)
>>> %timeit set(map(lambda x: x**2, range(10)))
3.72 µs ± 207 ns per loop (mean ± std. dev. of 7 runs, 100000 loops each)
>>> %timeit {el**2 for el in range(10)}
3.11 µs ± 309 ns per loop (mean ± std. dev. of 7 runs, 100000 loops each)
On top of that, comprehensions can be nested, use if statements (thus, replace the filter function), and operate on different data structures. In the following example, we use a comprehension to run over a list of dictionaries, and create a new list that contains character names, but only for characters with an age that is below 15 (only they can go to Neverland, you know):
>>> characters = [
{'name': 'Peter Pan', 'age': 13, 'type': 'boy'},
{'name': 'Wendy Darling', 'age': 14, 'type': 'girl'},
{'name': 'Captain Cook', 'age': 45, 'type': 'pirate'}
# just guessing
]
>>> [el['name'] for el in characters if el['age'] < 15]
['Peter Pan', 'Wendy Darling']
We can even use the comprehension to swap keys and values of the dictionary that way. In the following code, we create a new dictionary by using the values of the existing one as keys, and by using keys as values:
D = {'A':1, 'B':2 }
{v:k for k, v in D.items()}
>>> {1:'A', 2:'B'}
This concludes our exposé into data structures. In the following chapters, we'll be using everything we just learned more extensively.