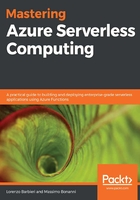
Azure Functions and PowerShell
Azure Functions support PowerShell, but the support for this language is in preview at the time of writing, so what we will say in this paragraph could change in the future.
The Azure Functions Runtime leverages the features of the PowerShell Core 6 (it is based on .NET Core and can run on Windows, macOS, and Linux) and includes native support for the new Azure AZ module (to interact with the Azure resources).
These features make Azure automation one of the ideal scenarios for using PowerShell within Azure Functions: you can react to an event produced by Azure (for example, a monitor alert) and run some operations on your resources.
When you create an Azure Function using PowerShell, you have all the benefits of the other languages you saw earlier in this chapter, and in particular, you have the following:
- Native bindings to respond to Azure monitoring alerts, resource changes through event grid, HTTP, or timer triggers, and more
- Portal and VS Code integration for authoring and testing scripts
- Integrated security to protect HTTP-triggered functions
- Support for hybrid connections and VNet to help manage hybrid environments
- The ability to run in an isolated local environment
You can create your first Azure Function with PowerShell in the same way you would with the other supported languages:
C:\MasteringServerless\myFirstPowershellFunction>func init --worker-runtime powershell MyFirstPowershellFunction
Writing profile.ps1
Writing requirements.psd1
Writing .gitignore
Writing host.json
Writing local.settings.json
Writing C:\MasteringServerless\myFirstPowershellFunction\.vscode\extensions.json
C:\MasteringServerless\myFirstPowershellFunction>func new --name HttpPowershellFunction --template HttpTrigger --language powershell
Select a template: HttpTrigger
Function name: [HttpTrigger] Writing C:\MasteringServerless\myFirstPowershellFunction\HttpPowershellFunction\run.ps1
Writing C:\MasteringServerless\myFirstPowershellFunction\HttpPowershellFunction\sample.dat
Writing C:\MasteringServerless\myFirstPowershellFunction\HttpPowershellFunction\function.json
The function "HttpPowershellFunction" was created successfully from the "HttpTrigger" template.
The folder structure for a PowerShell function is shown in the following diagram:

The run.ps1 file inside the folder called as the function contains the PowerShell code for the function itself:
using namespace System.Net
# Input bindings are passed in via param block.
param($Request, $TriggerMetadata)
# Write to the Azure Functions log stream.
Write-Host "PowerShell HTTP trigger function processed a request."
# Interact with query parameters or the body of the request.
$name = $Request.Query.Name
if (-not $name) {
$name = $Request.Body.Name
}
# Analyzes the request and create the response
# ..... look at the github for the complete code
# Associate values to output bindings by calling 'Push-OutputBinding'.
Push-OutputBinding -Name Response -Value ([HttpResponseContext]@{
StatusCode = $status
Body = $body
})
In this case, the triggers and bindings are passed as the param definition, and you can write the log using the standard Write-Host cmdlet.
The triggers and bindings definitions, as usual, are in the function.json file with a similar format to the other languages.
The PowerShell language worker can automatically manage the integration of the Azure modules without you needing to reference them in the configuration files (this feature demonstrates that PowerShell in Azure Functions was created with Azure automation in mind). You can enable the integration in the host file by setting the managedDependency property to true:
{
"version": "2.0",
"managedDependency": {
"Enabled": "true"
}
}
The worker also takes care of the critical and security updates on the Azure modules, and you can enable this by setting the Az property in the requirements.psd1 file:
@{
Az = '1.*'
}
Finally, the profile.ps1 file is used by the runtime to automatically authenticate the Azure Function host to the Azure services. profile.ps1 is run when the function app starts, and you can add the common commands to execute for each PowerShell functions in your project in that file:
# Azure Functions profile.ps1
#
# This profile.ps1 will get executed every "cold start" of your Function App.
# "cold start" occurs when:
#
# * A Function App starts up for the very first time
# * A Function App starts up after being de-allocated due to inactivity
#
# You can define helper functions, run commands, or specify environment variables
# NOTE: any variables defined that are not environment variables will get reset after the first execution
# Authenticate with Azure PowerShell using MSI.
# Remove this if you are not planning on using MSI or Azure PowerShell.
if ($env:MSI_SECRET -and (Get-Module -ListAvailable Az.Accounts)) {
Connect-AzAccount -Identity
}
# Uncomment the next line to enable legacy AzureRm alias in Azure PowerShell.
# Enable-AzureRmAlias
# You can also define functions or aliases that can be referenced in any of your PowerShell functions.
Once you create your function project, you can run the functions using the following command:
C:\MasteringServerless\myFirstPowershellFunction>func start host
Next, we will be looking at language extensibility.