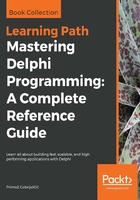
Optimization
The Optimization option controls code optimization. The possible values are On and Off. This setting can also be controlled with compiler directives {$OPTIMIZATION ON} and {$OPTIMIZATION OFF} or with equivalent {$O+} and {$O-}.
When optimization is enabled, the compiler optimizes generated code for speed. Optimizations are guaranteed not to change the behavior of the program. The only reason you may want to turn optimization off is to improve debugging. When optimization is turned on, debugging—especially stepping through the code and evaluating variables—may not be working correctly in all cases.
The $O (or $OPTIMIZATION) directive can only turn optimization on or off for an entire method. You cannot turn optimization on or off for selected lines within a method.
To show the effect of different compiler options, I have written a CompilerOptions demo. When you click the Optimization button, the following code will be run twice—once with optimization turned on and once off.
The code initializes an array and then does some calculations on all of its elements. All of this is repeated 1,000 times because otherwise it is hard to measure differences. Modern CPUs are fast!
function NonOptimized: int64;
var
arr1: array [1..50000] of Integer;
i,j: Integer;
begin
for j := 1 to 1000 do
begin
for i := Low(arr1) to High(arr1) do
arr1[i] := i;
for i := Low(arr1) to High(arr1)-1 do
arr1[i] := arr1[i] + arr1[i+1];
end;
end;
The results will be different depending on the CPU speed and model, but on all computers the optimized version should be significantly faster. On my test machine, the optimized version runs approximately six times faster than the non-optimized! Here are the results:

Although you can turn compilation options on and off with compiler directives, Delphi provides no direct support for switching back to the previous state. You can, however, check whether a compilation option is currently turned on or off by using the {$IFOPT} compiler directive.
Then you can define or undefine some conditional compilation symbol that will tell you later what the original state of the compilation option was:
{$IFOPT O+}{$DEFINE OPTIMIZATION}{$ELSE}{$UNDEF OPTIMIZATION}{$ENDIF}
{$OPTIMIZATION ON}
When you want to restore the original state, use this conditional to turn the compilation option on or off:
{$IFDEF OPTIMIZATION}{$OPTIMIZATION ON}{$ELSE}{$OPTIMIZATION OFF}{$ENDIF}
This technique is used throughout the CompilerOptions demo with different compilation options.