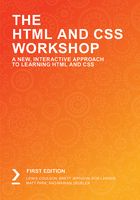
Structuring an HTML Document
A requirement of HTML5 is that all HTML documents are structured with a root element, the html element, and two child elements, the head element, and the body element.
HTML
The html element is the root element of an HTML document. In an HTML document, the only thing that appears outside the html element is the doctype declaration, which appears above the html element. Before we put any content on our web page, the code looks like this:
<!doctype html>
<html lang="en"></html>
The html element is the top-level element and all other elements must be a descendant of it. The html element has two children – one head element and a body element, which must follow the head element.
It is strongly recommended that you add a lang attribute to your html element to allow the browser, screen readers, and other technologies, such as translation tools, to better understand the text content of your web page.
Head
The head element contains metadata. It stores information about the HTML document that is used by machines – browsers, web crawlers, and search engines – to process the document. This section of an HTML document is not rendered for human users to read.
The minimum content the head element can have is a title element. Within a web page, the code would look like this:
<!doctype html>
<html lang="en">
<head>
<title>Page Title</title>
</head>
</html>
Body
An HTML element is expected to have one body element and it is expected to be the second child of the html element following the head element.
Our minimal HTML document would therefore have the following code:
<!doctype html>
<html lang="en">
<head>
<title>Page Title</title>
</head>
<body>
</body>
</html>
The body element represents the content of the document. Not everything in the body will necessarily be rendered in the browser, but all human-readable content should be hosted in the body element. This includes headers and footers, articles, and navigation.
You will learn more about the elements that can be the content of the body element throughout the following chapters.
Our First Web Page
In our first example, we will create a very simple web page. This will help us to understand the structure of an HTML document and where we put different types of content.
Exercise 1.01: Creating a Web Page
In this exercise, we will create our first web page. This is the foundation upon which the future chapters will build.
The steps are as follows:
- To start, we want to create a new folder, named chapter_1, in a directory of your choice. Then open that folder in Visual Studio Code (File > Open Folder…).
- Next, we will create a new plain text file by clicking File > New File. Then, save it in HTML format, by clicking File > Save As...and enter the File name: index.html. Finally, click on Save.
- In index.html, we start by writing the doctype declaration for HTML5:
<!DOCTYPE html>
- Next, we add an HTML tag (the root element of the HTML document):
<html lang="en">
</html>
- In between the opening and closing tags of the html element, we add a head tag. This is where we can put metadata content. For now, the head tag will contain a title:
<head>
<title>HTML and CSS</title>
</head>
- Below the head tag and above the closing html tag, we can then add a body tag. This is where we will put the majority of our content. For now, we will render a heading and a paragraph:
<body>
<h1>HTML and CSS</h1>
<p>How to create a modern, responsive website with HTML and CSS</p>
</body>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the following web page in your browser:

Figure 1.10: The web page as displayed in the Chrome web browser
Metadata
The head element is home to most machine-read information in an HTML document. We will look at some commonly used metadata elements and how they enhance a web page and how they can optimize a web page for search engines and modern browsers.
The following elements are considered metadata content: base, link, meta, noscript, script, style, and title.
We've already added a title element in the previous exercise. This is the name of your web page and it appears in the tab of most modern browsers as well as in a search engine's results as the heading for the web page's listing.
The link element lets us determine the relationships between our document and external resources. A common use for this element is to link to an external style sheet. We will look at that use case in the section on CSS later in this chapter. There are several other uses for the link element. These include linking to icons and informing the browser to preload assets.
The base element lets you set a base URL. This will be used as the base for all relative URLs in the HTML document. For example, we could set the base href and then link to a style sheet with a relative URL:
<base href="http://www.example.com">
<link rel="stylesheet" href="/style.css">
This would result in our browser trying to download a style sheet from http://www.example.com/style.css.
The meta element acts as a catch-all for other metadata not represented by the other metadata content elements. For example, we can use the meta element to provide a description or information about the author of the web page.
Another use for the meta element is to provide information about the HTML document, such as the character encoding used. This can be very important as text characters will render differently or not at all if not set correctly. For example, we normally set the character encoding to UTF-8:
<meta charset="utf-8">
This character encoding declaration tells the browser the character set of the document. UTF-8 is the default and is recommended. This gives information to the browser but does not ensure the document conforms to the character encoding. It is also necessary to save the document with the correct character encoding. Again, UTF-8 is often the default but this varies with different text editors.
It is important that the character encoding declaration appears early in the document as most browsers will try to determine the character encoding from the first 1,024 bytes of a file. The noindex attribute value is set for the web pages that need not be indexed, whereas the nofollow attribute is set for preventing the web crawler from following links.
Another meta element that is very useful for working with mobile browsers and different display sizes is the viewport element:
<meta name="viewport" content="width=device-width, initial-scale=1">
The viewport element is not standard but is widely supported by browsers and will help a browser define the size of the web page and the scale to which it is zoomed on smaller display sizes. The units of viewport height and viewport width are vh and vw respectively; for example, 1vh = 1% of the viewport width. We will dive deeper into the viewport element and other aspects of responsive web development in Chapter 6, Responsive Web Design and Media Queries.
The script element lets us embed code in our HTML document. Typically, the code is JavaScript code, which will execute when the browser finishes parsing the content of the script element.
The noscript element allows us to provide a fallback for browsers without scripting capabilities or where those capabilities are switched off by the user.
We will look at the style element in more detail when we look at CSS later in this chapter.
These elements won't appear on the web page as content the user sees in the browser. What they do is give web developers a lot of power to tell a browser how to handle the HTML document and how it relates to its environment. The web is a complex environment and we can describe our web page for other interested parties (such as search engines and web crawlers) using metadata.
Exercise 1.02: Adding Metadata
In this exercise, we will add metadata to a web page to make it stand out in search engine results. The page will be a recipe page for a cookery website called Cook School. We want the page's metadata to reflect both the importance of the individual recipe and the larger website so it will appear in relevant searches.
To achieve this, we will add metadata – a title, a description, and some information for search engine robots. On the web, this information could then help users find a blog post online via a search engine.
Here are the steps we will follow:
- Open the chapter_1 folder in VSCode (File > Open Folder…) and create a new plain text file by clicking File > New File. Then, save it in HTML format by clicking File > Save As...and enter the File name: metadata.html. Next, we will start with a basic HTML document:
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Metadata will go in the head -->
</head>
<body>
<!-- Cupcake recipe will go in the body -->
</body>
</html>
- Let's add a title for the recipe page that will be relevant to users who have navigated to the page or who are looking at a search engine results page. We will add this to the head element:
<title>Cook School - Amazing Homemade Cupcakes</title>
- Just after the opening <head> element, we will add a metadata element, <meta>, to let the browser know which character encoding to use:
<meta charset="utf-8">
- Next, we are going to add a description meta element below the title element:
<meta name="description" content="Learn to bake delicious, homemade cupcakes with this great recipe from Cook School.">
- We will add another meta element. This time, it is the robots meta element, which is used to make search engine crawling and indexing behave in a certain way. For example, if you didn't want a page to be indexed by a search engine, you could set the value to noindex. We will set a value of nofollow, which means a web crawler will not follow links from the page:
<meta name="robots" content="nofollow">
If you don't set this tag, the default value will be index and follow. This is normally what you want but you might not want a search engine to follow links in comments or index a particular page.
- The viewport meta element, which is very useful for working with mobile browsers and different display sizes, is added just below the title element in the head element:
<meta name="viewport" content="width=device-width, initial-scale=1">
- To finish, let's add some content to the body element that correlates with the metadata we've added:
<h1>Cook School</h1>
<article>
<h2>Amazing Homemade Cupcakes</h2>
<p>Here are the steps to serving up amazing cupcakes:</p>
<ol>
<li>Buy cupcakes from a shop</li>
<li>Remove packaging</li>
<li>Pretend you baked them</li>
</ol>
</article>
If you now right-click on the filename in VSCode on the left-hand side of the screen and select open in default browser, you will see the following web page in your browser:

Figure 1.11: The web page as displayed in the Chrome web browser
The important thing in the relationship between content and metadata is that they should make sense together. There is no point in adding keywords or writing a description of cars if the article is about cupcakes. The metadata should describe and relate to your actual content.
There are many search engines out there and they all do things a bit differently, and often with their own bespoke meta elements. If you would like to know more about how Google's search engine understands the meta tags from your web page's source code, some information is available at https://packt.live/35fRZ0F.