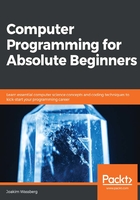
Syntax and the building blocks of a programming language
Just as human languages have grammar to dictate the rules of the language, a programming language has syntax. The syntax is the rules for how we write a program using a language. There is one big difference between grammar and syntax and that is about forgiveness for errors. If you meet someone who speaks your native language but makes some errors here and there, you will still be able to understand what that person is trying to communicate to you. That is not the case for the syntax of a programming language. It does not forgive at all, and you will need to get it spot on:

Figure 2.7: Humans understand each other even if the grammar is wrong
As we discussed earlier, the code we write will be translated by either a compiler or an interpreter, and for that translation to work, the syntax must be flawless.
Each programming language has its own syntax rules, but as we saw in the family tree earlier, languages can be related. So, many languages share a syntax with only slight variations, where others have a more specialized syntax. When learning a new language, we must learn the syntax for that language. That is why moving between closely related languages is easier as they will most likely share a lot of their syntax.
If we have an error in the syntax, it will be discovered during the translation, and here is where a compiled and an interpreted language will differ. For a compiled language, all the translation will be done before we can execute the program. If we have an error in the syntax, the compilation will stop as soon as the compiler discovers the mistake. We must then find the fault and correct it, then let the compiler try to translate the code again. It is not until our code does not have any syntax errors that we have something we can run completely:

Figure 2.8: A compiler will not produce any output until there are no errors in the syntax
This is different for an interpreted language as it will translate line by line as we run the program. This means that a syntax error can be hidden in a corner of the program that is rarely executed and will not be discovered until we eventually want to run that line of code. When this happens, the program will crash with an error message letting us know what problem was there with our syntax:

Figure 2.9: An interpreter will translate every line it encounters and executes it until it finds a syntax error
This means that a source code document that we have written can either be syntactically correct or incorrect. The syntax is a set of rules defining how the source code will be written and structured. But that is not all. The syntax also defines other things, such as the words that make up the language. These are called keywords.
Keywords
When learning a new language, we must keep track of its keywords as these words are reserved by the language and so we can't use them to name things in our program. If we use a keyword by accident for something other than its intended use, we will get an error. Keywords are sometimes also referred to as reserved words.
A language will typically have between 30 and 50 keywords. Here is a list of some common keywords in many languages:
- for
- if
- else
- break
- continue
- return
- while
Most programming languages are case sensitive, so the use of uppercase and lowercase letters matters—for example, if is not the same thing as If or IF.
Apart from keywords, we also have something called operators, which we can use to represent the actions we want to perform on data.
Operators
A programming language will also have several operators, and these are what we use to accomplish things such as addition and multiplication, as well as to compare items. The symbols that can be used are also defined as part of the language syntax. Here is a list of some commonly used operators:

Table 2.1 – Commonly used operators in programming languages
Operators are so-called because they perform operations on data. As we can see in the preceding table, there are operators to perform arithmetic operations, such as addition and multiplication. Other operators are used for comparison—for example, to see whether two values are equal, whether one is greater than another, and so on. In Chapter 6, Working with Data – Variables we will see more about what operators are typically found in a programming language and how they can be used.
Having our code in one long sequence would make it difficult to read. It would be like having a book with no chapters or paragraphs. To add the concept of chapters and paragraphs to our code, we use something called code blocks.
Code blocks
It is common for a language to also allow us to define blocks of code. There are several reasons why you would want to do that, and we will talk more about them in later chapters. However, for now, we can think of a block of code like a paragraph in standard text. The language then defines how we mark the beginning and end of the block. A common technique that many languages employ is using parentheses, also called braces or curly brackets—{}. Everything within these parentheses is considered part of the block. Other languages might have different ways to do the same thing, so again, when switching between languages, we must learn what the syntax rules are for that language.
Now that we have covered some of the basic concepts that a programming language uses to define its syntax, we should make one clarification. Many concepts that come up in programming share names with concepts in mathematics. So, let's see how programming is related to mathematics.
Relations to mathematics
Programming is closely related to mathematics as programming has borrowed many concepts from it. One of these concepts is the use of variables. In Chapter 6, Working with Data – Variables, we will talk about what a variable is and how it works, but they are essentially the same as they are in mathematics in the sense that we can use a name to represent a variable (a value that can change). The rules for how we can name variables are also part of the language syntax.
Another concept borrowed from mathematics is functions. In mathematics, a function is something that takes an input value and transforms it in some way to produce an output. This is close to how we can describe functions in programming as well, but that is not all there is to functions in programming. We will talk about functions in Chapter 8, Understanding Functions, and then we will see that we need to think about programming functions in a different way than how we view their mathematical equivalent.
One thing we must remember when approaching programming is that if we understand how these concepts work in mathematics, that does not mean that we can apply this knowledge directly to programming, even if they happen to share the same name. They will be related, but how things are done in programming will differ from how things work in math.