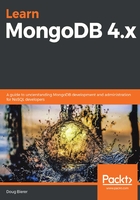
Connecting with the mongo shell
After installing MongoDB on a server, a separate executable named mongo is also installed. Here is an example of opening the shell without any parameters from the Docker container mentioned at the start of this chapter:

From this prompt, you are now able to view database information and query collections and perform other vital database operations.
The mongo shell command-line switches
There are a number of command-line switches associated with the mongo shell (https://docs.mongodb.com/manual/mongo/#the-mongo-shell), the most important of which are summarized here. You should note that the mongo shell is used to connect to a mongod server instance, which is typically running as a system daemon or service.
The following table presents a summary of the more important mongo shell command-line switches:

All of the command-line switches summarized in the preceding table can be mixed together. Here is an example that connects to a remote mongod instance on IP address 192.168.2.57, which listens on port 28028. The user doug is authenticated using the security database. The connection is secured by TLS. Have a look at the following code snippet:
mongo --host 192.168.2.57:28028 --username doug \
--authenticationDatabase security --tls
mongo mongodb://doug@192.168.2.57:28028/?authSource=security&tls=true.
Customizing the mongo shell
There are a certain number of customization options you can perform on the mongo shell. Simply by creating a .mongorc.js file (https://docs.mongodb.com/manual/reference/program/mongo/#mongo-mongorc-file) in your user home directory, you can customize the prompt to display dynamic information such as the uptime, hostname, database name, number of commands executed, and so forth. This is accomplished by defining a JavaScript function to the prompt reserved variable name, whose return value is echoed to form the prompt.
Here is a sample .mongorc.js script that displays the MongoDB version, hostname, database name, uptime, and command count:
host = db.serverStatus().host;
cmd = 1;
prompt = function () {
upt = db.serverStatus().uptime;
dbn = db.stats().db;
return "\nMongoDB " + host + "@" + dbn
+ "[up:" + upt + " secs]\n" + cmd++ + ">";
}
By placing this file in the user home directory, subsequent use of the mongo command will display the modified prompt, as shown:

Let's now look at the shell command helpers.
Shell command helpers
When using the mongo shell interactively, there is a handy set of shell command helpers that facilitate discovery and navigation while in the shell. The command helpers are summarized here:

In the following example, we use shell helper commands to get a list of databases, use the admin database, and list collections in this database:

Let's now learn to use the mongo shell methods.
Using mongo shell methods
The mongo shell makes available a rich set of shell methods that include the following categories:

In this chapter, we will focus on basic database, collection, cursor, and monitoring methods. In other chapters in this book, we will cover user and role management, replication, and sharding methods. Examples of shell methods that allow you to perform common database operations are covered in the next section of this chapter.
Running scripts using the shell
You can use the mongo shell to run scripts that use JavaScript syntax. This is a good way to permanently save complex shell commands or perform bulk operations. There are two ways to run JavaScript-based scripts using the mongo shell: directly from the command line, or from within the mongo shell.
Running a script from the command line
Here is an example of running a shell script that inserts documents into the sweetscomplete database from the command line:
mongo /path/to/repo/sample_data/sweetscomplete_customers_insert.js
Here is the resulting output:

Let's now run a script from inside the shell.
Running a script from inside the shell
You can also run a shell script from inside the mongo shell using the load(<filename.js>) command helper. After having first returned to the mongo shell, issue the use sweetscomplete; command. You can run the sweetscomplete_products_insert.js script that is located in the /path/to/repo/sample_data directory, as illustrated in the following code snippet:
mongo --quiet
use sweetscomplete;
show collections;
load("/path/to/repo/sample_data/sweetscomplete_products_insert.js");
show collections;
When you examine the output from this command next, note that you first need to use the database. We then look at the list of collections before and after running the script. Notice in the following screenshot that a new products collection has been added:

Let's now look at the shell script syntax.
Shell script syntax
It's very important to understand that you cannot use shell command helpers from an external JavaScript script, even if you run the script from inside the shell! Many of the shell methods you may wish to use reference the db object. When you are running shell methods from inside the shell, this is not a problem: when you issue the use command, this anchors the db object to the database currently in use. Since the use command is a shell command helper, however, it cannot be used inside an external script. Accordingly, the first set of commands you need to add to an external shell script is needed to set the db object to the desired database.
This can be accomplished as follows, where <DATABASE> is the name of the target database you wish to operate upon:
conn = new Mongo();
db = conn.getDB("<DATABASE>");
From this point on, you can use the db object as you would with any other shell method. Here is a sample shell script that assigns the db object to the sweetscomplete database, removes the common collection, and then inserts a document:
conn = new Mongo();
db = conn.getDB("sweetscomplete");
db.common.drop();
db.common.insertOne(
{
"key" : "gender",
"data" : { "M" : "male", "F" : "female" }
});
We now turn our attention to common database operations: inserting, updating, deleting, and querying.