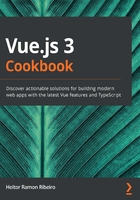
Creating your first TypeScript Vue component with vue-class-component
As Vue components are object-based and have a strong relationship with the this keyword of the JavaScript object, it gets a little bit confusing to develop a TypeScript component.
The vue-class-component plugin uses the ECMAScript decorators proposal to pass the statically typed values directly to the Vue component and makes the process of the compiler understand what is happening more easily.
Getting ready
The pre-requisite for this recipe is as follows:
- Node.js 12+
The Node.js global objects that are required are as follows:
- @vue/cli
- @vue/cli-service-global
How to do it...
First, we need to create our Vue CLI project. We can use the one we created in the last recipe or start a new one. To find how to create a Vue CLI project with TypeScript, please check the 'Adding TypeScript to a Vue CLI project' recipe.
Follow the instructions to create your first Vue component with Typescript and vue-class-component:
- Create a new file inside the src/components folder, called Counter.vue.
- Now, let's start making the script part of the Vue component. We will make a class that will have data with a number, two methods—one for increasing and another for decreasing—and, finally, a computed property to format the final data:
<script lang="ts">
import Vue from 'vue';
import Component from 'vue-class-component';
@Component
export default class Counter extends Vue {
valueNumber: number = 0;
get formattedNumber() {
return `Your total number is: ${this.valueNumber}`;
}
increase() {
this.valueNumber += 1;
}
decrease() {
this.valueNumber -= 1;
}
}
</script>
- It's time to create the template and rendering for this component. The process is the same as a JavaScript Vue file. We will add the buttons for increasing and decreasing the value and showing the formatted text:
<template>
<div>
<fieldset>
<legend>{{ formattedNumber }}</legend>
<button @click="increase">Increase</button>
<button @click="decrease">Decrease</button>
</fieldset>
</div>
</template>
- In the App.vue file, we need to import the component we just created:
<template>
<div id="app">
<Counter />
</div>
</template>
<script lang="ts">
import { Component, Vue } from 'vue-property-decorator';
import Counter from './components/Counter.vue';
@Component({
components: {
Counter,
},
})
export default class App extends Vue {
}
</script>
<style lang="stylus">
#app
font-family 'Avenir', Helvetica, Arial, sans-serif
-webkit-font-smoothing antialiased
-moz-osx-font-smoothing grayscale
text-align center
color #2c3e50
margin-top 60px
</style>
- Now, when you run the npm run serve command on Terminal (macOS or Linux) or Command Prompt/PowerShell (Windows), you will see your component running and executing on screen:
How it works...
The vue-class-component plugin makes use of the new proposal of decorators to inject and pass some attributes to the classes on TypeScript.
This injection helps in the process of simplifying the development of a component with a syntax more aligned with TypeScript than with the Vue common object.
See also
Find more information about vue-class-component at https://github.com/vuejs/vue-class-component.