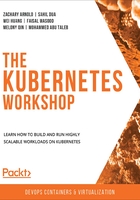
Common kubectl Commands
As previously described, kubectl is a CLI tool that is used to communicate with the Kubernetes API server. kubectl has a lot of useful commands for working with Kubernetes. In this section, we're going to walk you through some commonly used kubectl commands and shortcuts that are used to manage Kubernetes objects.
Frequently Used kubectl Commands to Create, Manage, and Delete Kubernetes Objects
There are several simple kubectl commands that you will use almost all the time. In this section, we will take a look at some of the basic kubectl commands:
- get <object>: You can use this command to get the list of the desired types of objects. Using all instead of specifying an object type will get the list of all kinds of objects. By default, this will get the list of specified object types in the default namespace. You can use the -n flag to get objects from a specific namespace; for example, kubectl get pod -n mynamespace.
- describe <object-type> <object-name>: You can use this command to check all the relevant information of a specific object; for example, kubectl describe pod mypod.
- logs <object-name>: You can use this command to check all the relevant logs of a specific object to find out what happened when that object was created; for example, kubectl logs mypod.
- edit <object-type> <object-name>: You can use this command to edit a specific object; for example, kubectl edit pod mypod.
- delete <object-type> <object-name>: You can use this command to delete a specific object; for example, kubectl delete pod mypod.
- create <filename.yaml>: You can use this command to create a bunch of Kubernetes objects that have been defined in the YAML manifest file; for example, kubectl create -f your_spec.yaml.
- apply <filename.yaml>: You can use this command to create or update a bunch of Kubernetes objects that have been defined in the YAML manifest file; for example, kubectl apply -f your_spec.yaml.
Walkthrough of Some Simple kubectl Commands
In this section, we're going to walk you through some of the commonly used kubectl commands. This section is mostly for demonstration purposes, so you may not see the exact output that you see in these images. However, this section will help you understand how these commands are used. You will use most of them extensively in later exercises, as well as throughout this book. Let's take a look:
- If you want to display nodes, use the following command:
kubectl get nodes
You will see an output similar to the following:
Figure 3.3: The output of kubectl get nodes command
Since we set up aliases in Exercise 3.01, Setting up Autocompletion, you can also get the same result using the following command:
k get no
- If you want to display all current namespaces, you can use the following command:
kubectl get namespaces
You should see an output similar to the following:
NAME STATUS AGE
default Active 7m5s
kube-node-lease Active 7m14s
kube-public Active 7m14s
kube-system Active 7m15s
You can also get the same result using the following shortened command:
k get ns
- If you want to check the version of kubectl, you can use the following command:
kubectl version
You will see an output similar to the following:
Client version: version.Info{Major:"1", Minor:"17", GitVersion:"v1.17.2, GitCommit: 59603c6e503c87169aea6106f57b9f242f64df89", GitTreeState:"clean", BuildDate:"2020-01-21T22:17:28Z, GoVersion:"go1.13.5", Compiler:"gc", Platform:"linux/amd64}
Server version: version.Info{Major:"1", Minor:"17", GitVersion:"v1.17.2, GitCommit: 59603c6e503c87169aea6106f57b9f242f64df89", GitTreeState:"clean", BuildDate:"2020-01-18T23:22:30Z, GoVersion:"go1.13.5", Compiler:"gc", Platform:"linux/amd64}
- If you want to see some information regarding your current Kubernetes cluster, you can use the following command:
kubectl cluster-info
You should see an output similar to the following:
Figure 3.4: The output of kubectl cluster-info command
Before we move on further with the demonstrations, we will mention a few commands that you can use to create a sample application, which we have already provided in the GitHub repository for this chapter. Use the following command to fetch the YAML specification for all the objects required to run the application:
curl https://raw.githubusercontent.com/PacktWorkshops/Kubernetes-Workshop/master/Chapter03/Activity03.01/sample-application.yaml --output sample-application.yaml
Now, you can deploy the sample-application.yaml file using the following command:
kubectl apply -f sample-application.yaml
If you can see the following output, this means that the sample application has been successfully created in your Kubernetes cluster:
deployment.apps/redis-back created
service/redis-back created
deployment.apps/melonvote-front created
service/melonvote-front created
Now that you have deployed the provided application, if you try any of the commands shown later in this section, you will see the various objects, events, and so on related to this application. Note that your output may not exactly match the images shown here:
- You can use the following command to get everything in your cluster under the default namespace:
kubectl get all
This will give an output similar to the following:
Figure 3.5: The output of kubectl get all command
- Events describe what has happened so far in the Kubernetes cluster, and you can use events to get a better insight into your cluster and aid in any troubleshooting efforts. To list all the events in the default namespace, use the following command:
kubectl get events
This will give an output similar to the following:
Figure 3.6: The output of kubectl get events command
- A service is an abstraction that's used to expose an application to the end-user. You will learn more about services in Chapter 8, Service Discovery. You can use the following command to list all services:
kubectl get services
This will give an output similar to the following:
Figure 3.7: The output of kubectl get services command
You can get the same result using the following shortened command:
k get svc
- A Deployment is an API object that allows us to easily manage and update pods. You will learn more about Deployments in Chapter 7, Kubernetes Controllers. You can get the list of Deployments using the following command:
kubectl get deployments
This should give a response similar to the following:
NAME READY UP-TO-DATE AVAILABLE AGE
aci-helloworld 1/1 1 1 34d
melonvote-front 1/1 1 1 7d6h
redis-back 1/1 1 1 7d6h
You can also get the same result using the following shortened version of the command:
k get deploy
Some Useful Flags for the get Command
As you have seen, the get command is a pretty standard command that is used when we need to get the list of objects in our cluster. It also has several useful flags. Let's take a look at a few of them here:
- If you want to list a particular type of resource from all your namespaces, you can add the --all-namespaces flag in the command. For example, if we want to list all Deployments from all namespaces, we can use the following command:
kubectl get deployments --all-namespaces
This will give an output similar to this:
Figure 3.8: The output of kubectl get deployments under all namespaces
You can also see that there is an additional column on the left-hand side that specifies the namespaces of the respective Deployments.
- If you want to list a specific type of resource from a specific namespace, you can use the -n flag. Here, the -n flag stands for namespace. For example, if you want to list all Deployments in a namespace called keda, the following command would be used:
kubectl get deployments -n keda
This command would show an output similar to the following:
Figure 3.9: The output of kubectl get deployments under the keda namespace
- You can add the --show-labels flag to display the labels of the objects in the list. For example, if you wanted to get the list of all the pods in the default namespace, along with their labels, you would use the following command:
kubectl get pods --show-labels
This command should give an output similar to the following:
Figure 3.10: The output of kubectl get pods with all labels
There is an additional column on the right-hand side that specifies the labels of the pods.
- You can use the -o wide flag to display more information about objects. Here, the -o flag stands for output. Let's look at a simple example of how to use this flag:
kubectl get pods -o wide
This will give an output similar to the following:
Figure 3.11: The output of kubectl get pods with additional information
You can also see there are additional columns on the right-hand side that specify which nodes the pods are running on, as well as the internal IP addresses of the node. You can find more ways to use the -o flag at https://kubernetes.io/docs/reference/kubectl/overview/#output-options.
Note
We will limit this section to commands that are commonly used to limit the scope of this chapter. You can find a lot more kubectl commands at https://kubernetes.io/docs/reference/generated/kubectl/kubectl-commands.