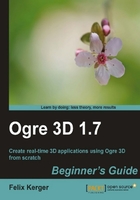
We will use the previous code, but create completely new code for the createScene()
function.
- Remove all code from the
createScene()
function. - First create an instance of
Sinbad.mesh
and then create a new scene node. Set the position of the scene node to (10,10,0), at the end attach the entity to the node, and add the node to the root scene node as a child:Ogre::Entity* ent = mSceneMgr->createEntity("MyEntity","Sinbad.mesh"); Ogre::SceneNode* node = mSceneMgr->createSceneNode("Node1"); node->setPosition(10,10,0); mSceneMgr->getRootSceneNode()->addChild(node); node->attachObject(ent);
- Again, create a new instance of the model, also a new scene node, and set the position to (10,0,0):
Ogre::Entity* ent2 = mSceneMgr->createEntity("MyEntity2","Sinbad.mesh"); Ogre::SceneNode* node2 = mSceneMgr->createSceneNode("Node2"); node->addChild(node2); node2->setPosition(10,0,0);
- Now add the following two lines to rotate the model and attach the entity to the scene node:
node2->pitch(Ogre::Radian(Ogre::Math::HALF_PI)); node2->attachObject(ent2);
- Do the same again, but this time use the function
yaw
instead of the functionpitch
and thetranslate
function instead of thesetPosition
function:Ogre::Entity* ent3 = mSceneMgr->createEntity("MyEntity3","Sinbad.mesh"); Ogre::SceneNode* node3 = mSceneMgr->createSceneNode("Node3",); node->addChild(node3); node3->translate(20,0,0); node3->yaw(Ogre::Degree(90.0f)); node3->attachObject(ent3);
- And the same again with
roll
instead ofyaw
orpitch:
Ogre::Entity* ent4 = mSceneMgr->createEntity("MyEntity4","Sinbad.mesh"); Ogre::SceneNode* node4 = mSceneMgr->createSceneNode("Node4"); node->addChild(node4); node4->setPosition(30,0,0); node4->roll(Ogre::Radian(Ogre::Math::HALF_PI)); node4->attachObject(ent4);
- Compile and run the program, and you should see the following screenshot:
We repeated the code we had before four times and always changed some small details. The first repeat is nothing special. It is just the code we had before and this instance of the model will be our reference model to see what happens to the other three instances we made afterwards.
In step 4, we added one following additional line:
node2->pitch(Ogre::Radian(Ogre::Math::HALF_PI));
The function pitch(Ogre::Radian(Ogre::Math::HALF_PI))
rotates a scene node around the x-axis. As said before, this function expects a radian as parameter and we used half of pi, which means a rotation of ninety degrees.
In step 5, we replaced the function call setPosition(x,y,z)
with translate(x,y,z)
. The difference between setPosition(x,y,z)
and translate(x,y,z)
is that setPosition
sets the position – no surprises here. translate
adds the given values to the position of the scene node, so it moves the node relatively to its current position. If a scene node has the position (10,20,30
) and we call setPosition(30,20,10)
, the node will then have the position (30,20,10
). On the other hand, if we call translate(30,20,10)
, the node will have the position (40,40,40
). It's a small, but important, difference. Both functions can be useful if used in the correct circumstances, like when we want to position in a scene, we would use the setPosition(x,y,z)
function. However, when we want to move a node already positioned in the scene, we would use translate(x,y,z)
.
Also, we replaced pitch(Ogre::Radian(Ogre::Math::HALF_PI))with yaw(Ogre::Degree(90.0f))
. The yaw()
function rotates the scene node around the y-axis. Instead of Ogre::Radian()
, we used Ogre::Degree()
. Of course, Pitch
and yaw
still need a radian to be used. However, Ogre 3D offers the class Degree()
, which has a cast operator so the compiler can automatically cast into a Radian()
. Therefore, the programmer is free to use a radian or degree to rotate scene nodes. The mandatory use of the classes makes sure that it's always clear which is used, to prevent confusion and possible error sources.
Step 6 introduces the last of the three different rotate function a scene node has, namely, roll()
. This function rotates the scene node around the z-axis. Again, we could use roll(Ogre::Degree(90.0f))
instead of roll(Ogre::Radian(Ogre::Math::HALF_PI))
.
The program when run shows a non-rotated model and all three possible rotations. The left model isn't rotated, the model to the right of the left model is rotated around the x-axis, the model to the left of the right model is rotated around the y-axis, and the right model is rotated around the z-axis. Each of these instances shows the effect of a different rotate function. In short, pitch()
rotates around the x-axis, yaw()
around the y-axis, and roll()
around the z-axis. We can either use Ogre::Degree(degree)
or Ogre::Radian(radian)
to specify how much we want to rotate.
- Which are the three functions to rotate a scene node?
a. pitch, yawn, roll
b. pitch, yaw, roll
c. pitching, yaw, roll