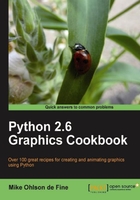
We now concentrate particularly on how the justification of the text in columns interacts with column anchor positions.
The following code contains a paragraph that is much too long to fit onto a single line. This is where we see how the term justify lets us decide whether we want the text to line up to the right of the column or to its left or perhaps even the center. The column width, in pixels, is specified and then the text is made to fit.
Run the following code and observe that the height of the column is only confined by the height of the canvas but the width, anchor position, justification, and font size determine how the text gets laid out on the canvas.
# justify_align_text_1.py #>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> from Tkinter import * root = Tk() root.title('North-south-east-west Placement with LEFT and RIGHT \ justification of Text') cw = 850 # canvas width ch = 720 # canvas height canvas_1 = Canvas(root, width=cw, height=ch, background="white") canvas_1.grid(row=0, column=1) orig_x = 220 orig_y = 20 offset_y = 20 jolly_text = "And now ladies and gentlemen she will attempt - for the very first time in the history of 17 Shoeslace street - a one handed right arm full toss teacup fling. Yes you lucky listners you are about to witness what, in recorded history, has never been attempted before without the aid of hair curlers and fluffy slippers." # width is maximum line length. #================================================================= # 1. Top-left (NE) ANCHOR POINT, no column justification specified. canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NE \ ,text="1. \ NORTH-EAST anchor, no column justification", fill='blue', width=200, \ font='Arial 10') canvas_1.create_text(orig_x, orig_y + 3 * offset_y, anchor = NE, \ text=jolly_text,\ fill='blue', width=150, font='Arial 10') #================================================================== # 2. Top-right (NW) ANCHOR POINT, no column justification specified. canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NW \ ,text="2. \ NORTH-WEST ancho, no column justification", fill='red', width=200, \ font='Arial 10') canvas_1.create_text(orig_x, orig_y + 3 * offset_y, anchor = NW, \ text= jolly_text,\ fill='red', width=200, font='Arial 10') #================================================================== orig_x = 600 canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NE \ ,text="3. \ SOUTH-EAST anchor, no column justification",fill='black', width=200, \ font='Arial 10') canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NW \ ,text="4. \ SOUTH-WEST anchor, no column justification", fill='#666666', \ width=200, font='Arial 10') #============================================================ orig_x = 600 orig_y = 280 # 3. BOTTOM-LEFT (SW) JUSTIFICATION, no column justification # specified. canvas_1.create_text(orig_x, orig_y + 2 * offset_y, anchor = SW, \ text=jolly_text,\ fill='#666666', width=200, font='Arial \ 10') #================================================================== # 4. TOP-RIGHT (SE) ANCHOR POINT, no column justification specified. canvas_1.create_text(orig_x, orig_y + 2 * offset_y, anchor = SE, \ text=jolly_text,\ fill='black', width=150, font='Arial 10') #=================================================================== orig_y = 350 orig_x = 200 # 5. Top-right (NE) ANCHOR POINT, RIGHT column justification # specified. canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NE , \ justify=RIGHT,\ text="5. NORTH-EAST anchor, justify=RIGHT", fill='blue', width=200, \ font='Arial 10 ') canvas_1.create_text(orig_x, orig_y + 3 * offset_y, anchor = NE, \ justify=RIGHT, \ text=jolly_text, fill='blue', width=150, font='Arial 10') #=================================================================== # 6. TOP-LEFT (NW) ANCHOR POINT, RIGHT column justification specified. canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NW \ ,text="6.\ NORTH-WEST anchor, justify=RIGHT", fill='red', width=200, \ font='Arial 10 ') canvas_1.create_text(orig_x, orig_y + 3 * offset_y, anchor = NW, \ justify=RIGHT,\ text=jolly_text, fill='red', width=200, font='Arial 10') #=================================================================== orig_x = 600 # Header lines for 7. and 8. canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NE \ ,text="7. \ SOUTH-EAST anchor, justify= CENTER", fill='black', width=160, \ font='Arial 10 ') canvas_1.create_text(orig_x, orig_y + 1 * offset_y, anchor = NW , \ text="8.\ SOUTH-WEST anchor, justify= CENTER", fill='#666666', width=200, \ font='Arial 10 ') #================================================================== orig_y = 600 # 7. TOP-RIGHT (SE) ANCHOR POINT, CENTER column justification # specified. canvas_1.create_text(orig_x, orig_y + 4 * offset_y, anchor = SE, \ justify= CENTER,\ text=jolly_text, fill='black', width=150, font='Arial 10') #=================================================================== # 8. BOTTOM-LEFT (SW) ANCHOR POINT, CENTER column justification # specified. canvas_1.create_text(orig_x, orig_y + 4 * offset_y, anchor = SW, \ justify= CENTER,\ text=jolly_text, fill='#666666', width=200, font='Arial 10') root.mainloop() #>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
The interaction of column width, anchor position, and justification are complex and the clearest way to explain the results is with annotated pictures of the canvas display resulting from execution. The following screenshot shows Top-right (NE) ANCHOR POINT, no justification specified (default LEFT justification).

The following screenshot shows the Top-right(SE)ANCHOR POINT, no justification specified:

The following screenshot shows the Bottom-right (SE) ANCHOR POINT, CENTER justification specified:
